C# Tutorial
C# examples, c# assignment operators, assignment operators.
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
Try it Yourself »
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:
Operator | Example | Same As | Try it |
---|---|---|---|
= | x = 5 | x = 5 | |
+= | x += 3 | x = x + 3 | |
-= | x -= 3 | x = x - 3 | |
*= | x *= 3 | x = x * 3 | |
/= | x /= 3 | x = x / 3 | |
%= | x %= 3 | x = x % 3 | |
&= | x &= 3 | x = x & 3 | |
|= | x |= 3 | x = x | 3 | |
^= | x ^= 3 | x = x ^ 3 | |
>>= | x >>= 3 | x = x >> 3 | |
<<= | x <<= 3 | x = x << 3 |


COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
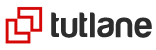
C# Assignment Operators with Examples
In c#, Assignment Operators are useful to assign a new value to the operand, and these operators will work with only one operand.
For example, we can declare and assign a value to the variable using the assignment operator ( = ) like as shown below.
If you observe the above sample, we defined a variable called “ a ” and assigned a new value using an assignment operator ( = ) based on our requirements.
The following table lists the different types of operators available in c# assignment operators.
Operator | Name | Description | Example |
---|---|---|---|
= | Equal to | It is used to assign the values to variables. | int a; a = 10 |
+= | Addition Assignment | It performs the addition of left and right operands and assigns a result to the left operand. | a += 10 is equals to a = a + 10 |
-= | Subtraction Assignment | It performs the subtraction of left and right operands and assigns a result to the left operand. | a -= 10 is equals to a = a - 10 |
*= | Multiplication Assignment | It performs the multiplication of left and right operands and assigns a result to the left operand. | a *= 10 is equals to a = a * 10 |
/= | Division Assignment | It performs the division of left and right operands and assigns a result to the left operand. | a /= 10 is equals to a = a / 10 |
%= | Modulo Assignment | It performs the modulo operation on two operands and assigns a result to the left operand. | a %= 10 is equals to a = a % 10 |
&= | Bitwise AND Assignment | It performs the Bitwise AND operation on two operands and assigns a result to the left operand. | a &= 10 is equals to a = a & 10 |
|= | Bitwise OR Assignment | It performs the Bitwise OR operation on two operands and assigns a result to the left operand. | a |= 10 is equals to a = a | 10 |
^= | Bitwise Exclusive OR Assignment | It performs the Bitwise XOR operation on two operands and assigns a result to the left operand. | a ^= 10 is equals to a = a ^ 10 |
>>= | Right Shift Assignment | It moves the left operand bit values to the right based on the number of positions specified by the second operand. | a >>= 2 is equals to a = a >> 2 |
<<= | Left Shift Assignment | It moves the left operand bit values to the left based on the number of positions specified by the second operand. | a <<= 2 is equals to a = a << 2 |
C# Assignment Operators Example
Following is the example of using assignment Operators in the c# programming language.
If you observe the above example, we defined a variable or operand “ x ” and assigning new values to that variable by using assignment operators in the c# programming language.
Output of C# Assignment Operators Example
When we execute the above c# program, we will get the result as shown below.
This is how we can use assignment operators in c# to assign new values to the variable based on our requirements.
Table of Contents
- Assignment Operators in C# with Examples
- C# Assignment Operator Example
- Output of C# Assignment Operator Example
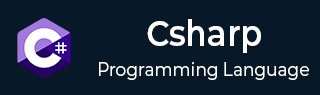
- C# Basic Tutorial
- C# - Overview
- C# - Environment
- C# - Program Structure
- C# - Basic Syntax
- C# - Data Types
- C# - Type Conversion
- C# - Variables
- C# - Constants
- C# - Operators
- C# - Decision Making
- C# - Encapsulation
- C# - Methods
- C# - Nullables
- C# - Arrays
- C# - Strings
- C# - Structure
- C# - Classes
- C# - Inheritance
- C# - Polymorphism
- C# - Operator Overloading
- C# - Interfaces
- C# - Namespaces
- C# - Preprocessor Directives
- C# - Regular Expressions
- C# - Exception Handling
- C# - File I/O
- C# Advanced Tutorial
- C# - Attributes
- C# - Reflection
- C# - Properties
- C# - Indexers
- C# - Delegates
- C# - Events
- C# - Collections
- C# - Generics
- C# - Anonymous Methods
- C# - Unsafe Codes
- C# - Multithreading
- C# Useful Resources
- C# - Questions and Answers
- C# - Quick Guide
- C# - Useful Resources
- C# - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
C# - Assignment Operators
There are following assignment operators supported by C# −
Operator | Description | Example |
---|---|---|
= | Simple assignment operator, Assigns values from right side operands to left side operand | C = A + B assigns value of A + B into C |
+= | Add AND assignment operator, It adds right operand to the left operand and assign the result to left operand | C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator, It subtracts right operand from the left operand and assign the result to left operand | C -= A is equivalent to C = C - A |
*= | Multiply AND assignment operator, It multiplies right operand with the left operand and assign the result to left operand | C *= A is equivalent to C = C * A |
/= | Divide AND assignment operator, It divides left operand with the right operand and assign the result to left operand | C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator, It takes modulus using two operands and assign the result to left operand | C %= A is equivalent to C = C % A |
<<= | Left shift AND assignment operator | C <<= 2 is same as C = C << 2 |
>>= | Right shift AND assignment operator | C >>= 2 is same as C = C >> 2 |
&= | Bitwise AND assignment operator | C &= 2 is same as C = C & 2 |
^= | bitwise exclusive OR and assignment operator | C ^= 2 is same as C = C ^ 2 |
|= | bitwise inclusive OR and assignment operator | C |= 2 is same as C = C | 2 |
The following example demonstrates all the assignment operators available in C# −
When the above code is compiled and executed, it produces the following result −
Operators in C#
Back to: C#.NET Tutorials For Beginners and Professionals
Operators in C# with Examples
What are operators in c#.
Operators in C# are symbols that are used to perform operations on operands. For example, consider the expression 2 + 3 = 5 , here 2 and 3 are operands , and + and = are called operators . So, the Operators in C# are used to manipulate the variables and values in a program.
Types of Operators in C#:
The Operators are classified based on the type of operations they perform on operands in C# language. They are as follows:
Arithmetic Operators in C#
Addition Operator (+): The + operator adds two operands. As this operator works with two operands, so, this + (plus) operator belongs to the category of the binary operator. The + Operator adds the left-hand side operand value with the right-hand side operand value and returns the result. For example: int a=10; int b=5; int c = a+b; //15, Here, it will add the a and b operand values i.e. 10 + 5
Subtraction Operator (-): The – operator subtracts two operands. As this operator works with two operands, so, this – (minus) operator belongs to the category of the binary operator. The Minus Operator substracts the left-hand side operand value from the right-hand side operand value and returns the result. For example: int a=10; int b=5; int c = a-b; //5, Here, it will subtract b from a i.e. 10 – 5
Modulus Operator (%): The % (Modulos) operator returns the remainder when the first operand is divided by the second. As this operator works with two operands, so, this % (Modulos) operator belongs to the category of the binary operator. For example: int a=10; int b=5; int c=a%b; //0, Here, it will divide 10 / 5 and it will return the remainder which is 0 in this case
Example to Understand Arithmetic Operators in C#:
In the below example, I am showing how to use Arithmetic Operators with Operand which are variables. Here, Num1 and Num2 are variables and all the Arithmetic Operators are working on these two variables.
In the following example, I am showing how to use Arithmetic Operators with Operand which are values. Here, 10 and 20 are values and all the Arithmetic Operators are working on these two values.
Assignment Operators in C#:
The Assignment Operators in C# are used to assign a value to a variable. The left-hand side operand of the assignment operator is a variable and the right-hand side operand of the assignment operator can be a value or an expression that must return some value and that value is going to assign to the left-hand side variable.
Simple Assignment (=):
Add assignment (+=):.
This operator is the combination of + and = operators. It is used to add the left-hand side operand value with the right-hand side operand value and then assign the result to the left-hand side variable. For example: int a=5; int b=6; a += b; //a=a+b; That means (a += b) can be written as (a = a + b)
Subtract Assignment (-=):
Multiply assignment (*=):.
This operator is the combination of * and = operators. It is used to multiply the left-hand side operand value with the right-hand side operand value and then assign the result to the left-hand side variable. For example: int a=10; int b=5; a *= b; //a=a*b; That means (a *= b) can be written as (a = a * b)
Division Assignment (/=):
This operator is the combination of / and = operators. It is used to divide the left-hand side operand value with the right-hand side operand value and then assign the result to the left-hand side variable. For example: int a=10; int b=5; a /= b; //a=a/b; That means (a /= b) can be written as (a = a / b)
Modulus Assignment (%=):
Example to understand assignment operators in c#:, relational operators in c#:.
The Relational Operators in C# are also known as Comparison Operators. It determines the relationship between two operands and returns the Boolean results, i.e. true or false after the comparison. The Different Types of Relational Operators supported by C# are as follows.
Equal to (==):
This Operator is used to return true if the left-hand side operand value is equal to the right-hand side operand value. For example, 5==3 is evaluated to be false. So, this Equal to (==) operator will check whether the two given operand values are equal or not. If equal returns true else returns false.
Not Equal to (!=):
Less than (<):.
This Operator is used to return true if the left-hand side operand value is less than the right-hand side operand value. For example, 5<3 is evaluated to be false. So, this Less than (<) operator will check whether the first operand value is less than the second operand value or not. If so, returns true else returns false.
Less than or equal to (<=):
Greater than (>):, greater than or equal to (>=):.
This Operator is used to return true if the left-hand side operand value is greater than or equal to the right-hand side operand value. For example, 5>=5 is evaluated to be true. So, this Greater than or Equal to (>=) operator will check whether the first operand value is greater than or equal to the second operand value. If so, returns true else returns false.
Example to Understand Relational Operators in C#:
Logical operators in c#:, logical or (||):.
This operator is used to return true if either of the Boolean expressions is true. For example, false || true is evaluated to be true. That means the Logical OR (||) operator returns true when one (or both) of the conditions in the expression is satisfied. Otherwise, it will return false. For example, a || b returns true if either a or b is true. Also, it returns true when both a and b are true.
Logical AND (&&):
Logical not ():.
This operator is used to return true if the condition in the expression is not satisfied. Otherwise, it will return false. For example, !a returns true if a is false.
Example to Understand Logical Operators in C#:
Bitwise operators in c#:, bitwise or (|), bitwise and (&):.
Bitwise OR operator is represented by &. This operator performs the bitwise AND operation on the corresponding bits of two operands involved in the operation. If both of the bits are 1, it gives 1. If either of the bits is not 1, it gives 0. For example, int a=12, b=25; int result = a&b; //8 How? 12 Binary Number: 00001100 25 Binary Number: 00011001 Bitwise AND operation between 12 and 25: 00001100 00011001 ======== 00001000 (it is 8 in decimal) Note : If the operands are of type bool, the bitwise AND operation is equivalent to the logical AND operation between them.
Bitwise XOR (^):
The bitwise OR operator is represented by ^. This operator performs a bitwise XOR operation on the corresponding bits of two operands. If the corresponding bits are different, it gives 1. If the corresponding bits are the same, it gives 0. For example, int a=12, b=25; int result = a^b; //21 How? 12 Binary Number: 00001100 25 Binary Number: 00011001 Bitwise AND operation between 12 and 25: 00001100 00011001 ======== 00010101 (it is 21 in decimal)
Example to Understand Bitwise Operators in C#:
In the above example, we are using BIT Wise Operators with integer data type and hence it performs the Bitwise Operations. But, if use BIT-wise Operators with boolean data types, then these bitwise operators AND, OR, and XOR behaves like Logical AND, and OR operations. For a better understanding, please have a look at the below example. In the below example, we are using the BIT-wise operators on boolean operands and hence they are going to perform the Logical AND, OR, and XOR Operations.
Unary Operators in C#:
The Unary Operators in C# need only one operand. They are used to increment or decrement a value. There are two types of Unary Operators. They are as follows:
Increment Operator (++) in C# Language:
Post increment operators:.
Syntax: Variable++; Example: x++;
Pre-Increment Operators:
Syntax: ++Variable; Example: ++x;
Decrement Operators in C# Language:
The Decrement Operator (–) is a unary operator. It takes one value at a time. It is again classified into two types. They are as follows:

Post Decrement Operators:
Pre-decrement operators:.
The Pre-Decrement Operators are the operators that are a prefix to its variable. It is placed before the variable. For example, –a will decrease the value of the variable a by 1.
Syntax: –Variable; Example: — x;
Note: Increment Operator means to increment the value of the variable by 1 and Decrement Operator means to decrement the value of the variable by 1.
Example to Understand Increment Operators in C# Language:
Example to understand decrement operators in c# language:, five steps to understand how the unary operators works in c#.
I see, many of the students and developers getting confused when they use increment and decrement operators in an expression. To make you understand how exactly the unary ++ and — operators work in C#, we need to follow 5 simple steps. The steps are shown in the below diagram.
Example to Understand Increment and Decrement Operators in C# Language:
Let us see one complex example to understand this concept. Please have a look at the following example. Here, we are declaring three variables x, y, and z, and then evaluating the expression as z = x++ * –y; finally, we are printing the value of x, y, and z in the console.
So, when you will execute the above program it will print the x, y, and z values as 11, 19, and 190 respectively.
Note: It is not recommended by Microsoft to use the ++ or — operators inside a complex expression like the above example. The reason is if we use the ++ or — operator on the same variable multiple times in an expression, then we cannot predict the output. So, if you are just incrementing the value of a variable by 1 or decrementing the variable by 1, then in that scenario you need to use these Increment or Decrement Operators. One of the ideal scenarios where you need to use the increment or decrement operator is inside a loop. What is a loop, why loop, and what is a counter variable, we will discuss this in our upcoming articles, but now just have a look at the following example, where I am using the for loop and increment operator?
Ternary Operator in C#:
The above statement means that first, we need to evaluate the condition. If the condition is true the first_expression is executed and becomes the result and if the condition is false, the second_expression is executed and becomes the result.
Example to understand Ternary Operator in C#:
Output: Result = 20
About the Author: Pranaya Rout
3 thoughts on “Operators in C#”
Leave a reply cancel reply.
- .NET Framework
- C# Data Types
- C# Keywords
- C# Decision Making
- C# Delegates
- C# Constructors
- C# ArrayList
- C# Indexers
- C# Interface
- C# Multithreading
- C# Exception
C# | Operators
Operators are the foundation of any programming language. Thus the functionality of C# language is incomplete without the use of operators. Operators allow us to perform different kinds of operations on operands . In C# , operators Can be categorized based upon their different functionality :
Arithmetic Operators
Relational Operators
Logical Operators
Bitwise Operators
Assignment Operators
Conditional Operator
In C#, Operators can also categorized based upon Number of Operands :
- Unary Operator: Operator that takes one operand to perform the operation.
- Binary Operator: Operator that takes two operands to perform the operation.
- Ternary Operator: Operator that takes three operands to perform the operation.
These are used to perform arithmetic/mathematical operations on operands. The Binary Operators falling in this category are :
- Addition: The ‘+’ operator adds two operands. For example, x+y .
- Subtraction: The ‘-‘ operator subtracts two operands. For example, x-y .
- Multiplication: The ‘*’ operator multiplies two operands. For example, x*y .
- Division: The ‘/’ operator divides the first operand by the second. For example, x/y .
- Modulus: The ‘%’ operator returns the remainder when first operand is divided by the second. For example, x%y .
The ones falling into the category of Unary Operators are:
- Increment: The ‘++’ operator is used to increment the value of an integer. When placed before the variable name (also called pre-increment operator), its value is incremented instantly. For example, ++x . And when it is placed after the variable name (also called post-increment operator ), its value is preserved temporarily until the execution of this statement and it gets updated before the execution of the next statement. For example, x++ .
- Decrement: The ‘- -‘ operator is used to decrement the value of an integer. When placed before the variable name (also called pre-decrement operator ), its value is decremented instantly. For example, – -x . And when it is placed after the variable name (also called post-decrement operator ), its value is preserved temporarily until the execution of this statement and it gets updated before the execution of the next statement. For example, x- – .
Relational operators are used for comparison of two values. Let’s see them one by one:
- ‘=='(Equal To) operator checks whether the two given operands are equal or not. If so, it returns true. Otherwise it returns false. For example, 5==5 will return true.
- ‘!='(Not Equal To) operator checks whether the two given operands are equal or not. If not, it returns true. Otherwise it returns false. It is the exact boolean complement of the ‘==’ operator. For example, 5!=5 will return false.
- ‘>'(Greater Than) operator checks whether the first operand is greater than the second operand. If so, it returns true. Otherwise it returns false. For example, 6>5 will return true.
- ‘<‘(Less Than) operator checks whether the first operand is lesser than the second operand. If so, it returns true. Otherwise it returns false. For example, 6<5 will return false.
- ‘>='(Greater Than Equal To) operator checks whether the first operand is greater than or equal to the second operand. If so, it returns true. Otherwise it returns false. For example, 5>=5 will return true.
- ‘<='(Less Than Equal To) operator checks whether the first operand is lesser than or equal to the second operand. If so, it returns true. Otherwise it returns false. For example, 5<=5 will also return true.
They are used to combine two or more conditions/constraints or to complement the evaluation of the original condition in consideration. They are described below:
- Logical AND: The ‘&&’ operator returns true when both the conditions in consideration are satisfied. Otherwise it returns false. For example, a && b returns true when both a and b are true (i.e. non-zero).
- Logical OR: The ‘||’ operator returns true when one (or both) of the conditions in consideration is satisfied. Otherwise it returns false. For example, a || b returns true if one of a or b is true (i.e. non-zero). Of course, it returns true when both a and b are true.
- Logical NOT: The ‘!’ operator returns true the condition in consideration is not satisfied. Otherwise it returns false. For example, !a returns true if a is false, i.e. when a=0.
In C#, there are 6 bitwise operators which work at bit level or used to perform bit by bit operations. Following are the bitwise operators :
- & (bitwise AND) Takes two numbers as operands and does AND on every bit of two numbers. The result of AND is 1 only if both bits are 1.
- | (bitwise OR) Takes two numbers as operands and does OR on every bit of two numbers. The result of OR is 1 any of the two bits is 1.
- ^ (bitwise XOR) Takes two numbers as operands and does XOR on every bit of two numbers. The result of XOR is 1 if the two bits are different.
- ~ (bitwise Complement) Takes one number as operand and invert each bits that is 1 to 0 and 0 to 1.
- << (left shift) Takes two numbers, left shifts the bits of the first operand, the second operand decides the number of places to shift.
- >> (right shift) Takes two numbers, right shifts the bits of the first operand, the second operand decides the number of places to shift.
Assignment operators are used to assigning a value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
- “=”(Simple Assignment) : This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
- “+=”(Add Assignment) : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
- “-=”(Subtract Assignment) : This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the current value of the variable on left from the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
- “*=”(Multiply Assignment) : This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
- “/=”(Division Assignment): This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
- “%=”(Modulus Assignment): This operator is combination of ‘%’ and ‘=’ operators. This operator first modulo the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a %= 2) = 0.
- “<<=”(Left Shift Assignment) : This operator is combination of ‘<<‘ and ‘=’ operators. This operator first Left shift the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a <<= 2) = 24.
- “>>=”(Right Shift Assignment) : This operator is combination of ‘>>’ and ‘=’ operators. This operator first Right shift the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a >>= 2) = 1.
- “&=”(Bitwise AND Assignment) : This operator is combination of ‘&’ and ‘=’ operators. This operator first “Bitwise AND” the current value of the variable on the left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a &= 2) = 2.
- “^=”(Bitwise Exclusive OR) : This operator is combination of ‘^’ and ‘=’ operators. This operator first “Bitwise Exclusive OR” the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a ^= 2) = 4.
- “|=”(Bitwise Inclusive OR) : This operator is combination of ‘|’ and ‘=’ operators. This operator first “Bitwise Inclusive OR” the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example :
If initially, value stored in a is 6. Then (a |= 2) = 6.
It is ternary operator which is a shorthand version of if-else statement. It has three operands and hence the name ternary. It will return one of two values depending on the value of a Boolean expression.
Explanation: condition: It must be evaluated to true or false. If the condition is true first_expression is evaluated and becomes the result. If the condition is false, second_expression is evaluated and becomes the result.
Please Login to comment...
Similar reads.
- CSharp Operators
- CSharp-Basics
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Why use the discard variable in C#?
When coding resharper recommends that if you're to discard or ignore the return of a method, that you use this syntax:
I know you could just call it without assignment to _ just the same, so why does the _ as an assignment matter?

- 8 Is very helpful for calls like TheMethodICouldCareLessAboutTheReturnValue(out _) or ( x, _, _) = TheMethodICouldCareLessAboutTheReturnValue – John Wu Commented Oct 28, 2021 at 14:24
- 32 @JohnWu TheMethodICouldntCareLessAboutTheReturnValue * ;) – Alexander Commented Oct 29, 2021 at 0:18
- 3 @Alexander: Things I don't care about get named things like asdasd , aaa , aaaa , and so on. TheMethodICouldCareLessAboutTheReturnValue implies some epsilon of caring, so the name is not entirely wrong. Maybe TheMethodICouldBarelyCareLessAboutTheReturnValue ? – Flater Commented Oct 29, 2021 at 8:22
- 1 "my epsilon on the scale of caring is usually zero" can be a useful thing to say outside of software engineering, too. – Cee McSharpface Commented Oct 29, 2021 at 18:01
5 Answers 5
It matters for two reasons. One is conventional, the other technical.
The conventional reason is that _ conveys active disinterest in the returned value. Sure, you could write var dontcare instead, but that's just a different arbitrary value.
But as you pointed out, you could also omit the assignment, so it's not just about choosing the shortest name possible. This brings us to the technical reason.
There are cases where you have to declare a parameter and you cannot simply omit it. This applies to out parameters in method calls, and named tuples when you don't care about all of the tuple's members.
There may be other use cases, these are the two I can think of because I encountered them before.
Is this necessary ? Well, it's an easy way to suppress warnings about unused variables. Not all developers care about warnings, but those who do would be pestered by these useless warnings for cases where they are knowingly not using a variable that the compiler forced them to declare anyway.

- (Also see my comment on Thomas's answer about repeated use of _ being magic) – Philip Kendall Commented Oct 28, 2021 at 15:07
- 1 Is it possible that the discard variable can save memory because since the value is going to be discarded, no memory needs to be allocated – CPlus Commented Oct 28, 2021 at 17:45
- 9 @user16217248 1) If the variable isn't used, the compiler can optimize it away anyway. 2) 8 bytes almost certainly isn't going to make a blind bit of difference to your program anyway. 3) Profile before you optimize. – Philip Kendall Commented Oct 28, 2021 at 17:50
- 6 (Warning: I'm nitpicking over semantics) I wouldn't say that using _ is "an easy way to suppress warnings about unused variables" - strictly speaking, to suppress a warning you use #pragma warning disable 01234 - whereas using a discard _ isn't "suppressing" a warning, it's about positively expressing your intent to ignore an unimportant or inconsequential result. The term "suppress" has connotations of basically sweeping issues under a rug (and invites future investigation during your next refactoring), whereas using discard is the opposite and implies finality and confidence. – Dai Commented Oct 28, 2021 at 22:53
- With tuples like (x,_)=expr you can almost say "we copied the tuple syntax from Python, so why not copy their successful style as well?". – Owen Reynolds Commented Oct 29, 2021 at 3:51
By using the discard _ , you are making it explicit that you, as the developer, understand that the method is returning a value but that you do not care about the value. This gives some insight into the code for reviews or future developers.
If you don't explicitly capture the return value, it is ambiguous as to your intention. Did you not realize that the method returns values? If you didn't realize that the method returned a value, does that also mean that you are missing a check against that return value against expectations? Or was it intentional and the return value doesn't mean anything in your context? These would be questions going through the mind of other developers that can easily be avoided with a very small change.

- 2 _ is slightly more than a standard variable name in that you can use it multiple times without error; var (_, _, foo) = bar; is valid while var (baz, baz, foo) = bar; is not. – Philip Kendall Commented Oct 28, 2021 at 15:06
- 1 @PhilipKendall Hm. I see the confusion. I mean "standard" in "a standard way of saying we are discarding this variable". The allowed reuse is a technical detail. You could prefix a variable with "discard" and end up with var (discard1, discard2, foo) , but there's no good reason to do that in a language like C# that lets you reuse the special _ name. Let me think on this wording a little bit. – Thomas Owens ♦ Commented Oct 28, 2021 at 15:12
- @ThomasOwens , just call it a discard, rather than a variable as that is what it is. – David Arno Commented Oct 28, 2021 at 17:07
- @PhilipKendall Unless you're using an earlier version of C# where _ is treated as a normal identifier and must be unique in a given lexical scope. Also, while you can have multiple separate _ discards inside a method, you cannot (unfortunately) have more than 1 discarded method parameter (which can happen when you're implementing an interface or using awkward out params). – Dai Commented Oct 28, 2021 at 22:57
- 1 @Dai have you got an example of cannot .. have more than 1 discarded method parameter ? – Caius Jard Commented Oct 29, 2021 at 15:53
Other answers have focussed on why the language includes the _ token, but I'd like to focus on why ReSharper is recommending it.
I think you are putting the emphasis on the wrong part of the message: ReSharper isn't asking you to add the _ , it's asking you to check whether the return value is important .
As the answer by Thomas Owens puts it:
If you didn't realize that the method returned a value, does that also mean that you are missing a check against that return value against expectations?
The tool is saying "Hey! This function returns a value! Maybe it's important!" If that return value is an error code, failing to handle it could lead to a serious bug - and one which you won't notice until the error condition comes up.
The suggestion to discard it with _ is a side-effect of that check: it's a way of answering the tool with a "Thanks for pointing it out, I've had a look, and in this case it's not important." It's making use of the language syntax so that you don't have to write an ugly "ignore check" comment next to the line.

- This was my first thought. I've always found the diagnostic messages for these sorts of issues somewhat misleading. It's not a syntax issue, it's a warning that you might be committing one of the classic blunders. – bta Commented Oct 29, 2021 at 21:48
- 1 So the compiler is combining two things: 1) "warning: unused return value", 2) "but I, the compiler still, think there's a 90% chance you actually want to ignore it so here's a quick way to turn off the warning I just gave you -- I have no opinion on whether it's good or bad style". – Owen Reynolds Commented Oct 30, 2021 at 22:49
I think it's simply a natural bias in C# editors towards suggesting newer features. I think that as discard (the underscore) was added in C#7.0, editor maintainers looked for possible new suggestions, saw _=exprWithIgnorableReturn(); as a possible use, and added that hint. I don't think there was any deeper thought process than that.
My reasoning is, as has been noted, we've long been able to use var dontCare=exprWithIgnorableReturn() , but choose not to. C# editor extensions could have had " uncaught possibly unneeded return value, consider using a dummy? ", but again, choose not to. The purpose of an ignoreable return value is that it can be ignored, as in completely ignored. Adding code to say "I realize this has a return value but choose to ignore it" is almost always clutter.
- "The purpose of an ignoreable return value is that it can be ignored" - is there some way of marking a return value as "ignorable"? Because if not this statement is meaningless, because all return values are ignorable as far as the language is concerned, but ignoring some is definitely a bug. – IMSoP Commented Oct 30, 2021 at 9:26
- @IMSoP The premise of the question is that there are actual useful functions where an ignorable return value is understood. They're run for the side-effect or to modify ref parameters, with a "maybe someone will want to see this" return value. You might ask the OP to give an example. Or a sample: stackoverflow.com/questions/2735701/… – Owen Reynolds Commented Oct 30, 2021 at 22:01
- I didn't say the functions don't exist , I asked if there was a way of marking those functions, or recognising them on sight, other than using the discard syntax. I also notice that at least two of the answers to the question you linked are discussing how to mark the value as ignored, either with comments, or with the equivalent in other languages of the _= syntax. – IMSoP Commented Oct 30, 2021 at 22:14
- @IMSoP I still feel as if the premise of the Q includes: 1) somehow a coder knows they want to ignore a return value (how is outside the scope of the Q) and 2) normally we don't mark that -- we call it like a non-value returning function. Number 2 is what my last line, about clutter, refers to. Igoring error values is a special case, which we clearly would comment. But I'm not sure how this line of questioning relates to what I wrote in the "answer" part. – Owen Reynolds Commented Oct 30, 2021 at 22:39
- 1 I disagree that it's clutter, but I also disagree with the premise of your answer that ReSharper is suggesting it just because it can. Some people don't think it's clutter, and do use comments and dummy variables to mark it in other languages; it's those people that the tool is trying to be helpful to. – IMSoP Commented Oct 31, 2021 at 23:40
Something else also to consider is that you also make it clear to the compiler that you do not care about this value.
- 6 Can you elaborate on how this helps the compiler to accomplish what goal? Perhaps with an example where not doing this results in suboptimal bytecode? – Philipp Commented Oct 29, 2021 at 8:17
- 1 But doesn't not capturing the return value, at all, make it equally clear to the compiler? It seems _=expr; only makes it more clear to us humans. – Owen Reynolds Commented Oct 29, 2021 at 22:10
- 1 Speaking as a human, it doesn't make it clearer to me either. It seems like a useless feature that compels the programmer to clutter his code with garbage. – trollkotze Commented Oct 31, 2021 at 18:12
Not the answer you're looking for? Browse other questions tagged c# .net or ask your own question .
- The Overflow Blog
- Ryan Dahl explains why Deno had to evolve with version 2.0
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
Hot Network Questions
- Pairing and structuring elements from a JSON array, with jq
- Do linguists have a noun for referring to pieces of commendatory language, as a sort of antonym of 'pejoratives'?
- Would it take less thrust overall to put an object into higher orbit?
- How old were Phineas and Ferb? What year was it?
- Why don't we observe protons deflecting in J.J. Thomson's experiment?
- Euler E152: "Analysin enim ineptam existimat"
- What is the rationale behind requiring ATC to retire at age 56?
- Is this misleading "convenience fee" legal?
- In Moon, why does Sam ask GERTY to activate a third clone before the rescue team arrives?
- How many people could we get off of the planet in a month?
- Population impacts on temperature
- Home water pressure higher than city water pressure?
- \includegraphics not reading \newcommand
- How to add a segment to an Excel radar plot
- How can I receive the responses to my broadcast requests through PF?
- In TNG: the Pegasus, why is Geordi in the first meeting with the Admiral?
- Not await an asynchronous method because it is like an endless loop - good practice?
- How to find intersecting linear equations between two lists?
- Is sudoku only one puzzle?
- Does the overall mAh of the battery add up when batteries are parallel?
- Is it OK to use the same field in the database to store both a percentage rate and a fixed money fee?
- What prevents applications from misusing private keys?
- What do all branches of Mathematics have in common to be considered "Mathematics", or parts of the same field?
- Should it be "until" or "before" in "Go home until it's too late"?
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
How does addition assignment operator behave
How does addition assignment operator behaves here -
- csharpcodeprovider
- i understand that x+= y means x = x + y, but what with the events – nicholas Commented Sep 30, 2015 at 6:55
- this is how you assign an event handler to btn.click event in c# – Kayani Commented Sep 30, 2015 at 6:59
- refer to: msdn.microsoft.com/en-us/library/aa645739(v=vs.71).aspx for more details – Kayani Commented Sep 30, 2015 at 7:00
- 1 This is what I typed in Google "c sharp += delegate" and what I have got under the second link C# - Delegates in section: "Multicasting of a Delegate" - Delegate objects can be composed using the "+" operator. A composed delegate calls the two delegates it was composed from. Only delegates of the same type can be composed. The "-" operator can be used to remove a component delegate from a composed delegate. Honestly, it's pure laziness. – Celdor Commented Sep 30, 2015 at 7:01
- is it what is called anonymous method? – nicholas Commented Sep 30, 2015 at 7:04
It adds an event handler to the event Click . When Click event is raised all the handlers method added to it are called.
For example:
And you add these methods to Click event like this:
When button is clicked the methods will be called in the order you added them, so the message box will be:
If you want specific info about += operator, MSDN says:
The += operator is also used to specify a method that will be called in response to an event; such methods are called event handlers. The use of the += operator in this context is referred to as subscribing to an event.
For more info look at:
https://msdn.microsoft.com/en-us/library/edzehd2t%28v=vs.110%29.aspx
http://www.dotnetperls.com/event
- it does wire the event handler to button's click, but it seems we can write code inline here – nicholas Commented Sep 30, 2015 at 7:12
- btn.Click += delegate(object sender, EventArgs e) { lblResult.Text = DateTime.Now.ToString(); }; – nicholas Commented Sep 30, 2015 at 7:12
- What you are talking about is Anonymous Methods , but they don't depend on events – Matteo Umili Commented Sep 30, 2015 at 7:15
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c# asp.net csharpcodeprovider or ask your own question .
- The Overflow Blog
- Ryan Dahl explains why Deno had to evolve with version 2.0
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
- What does a new user need in a homepage experience on Stack Overflow?
Hot Network Questions
- What is the rationale behind requiring ATC to retire at age 56?
- Fitting 10 pieces of pizza in a box
- Bending moment equation explanation
- Can I remove duplicate capacitors while merging two modules?
- Comparing the Order of STFT and Windowing in Audio Data Preprocessing for Machine Learning: Which Approach is Better?
- Is it OK to use the same field in the database to store both a percentage rate and a fixed money fee?
- Is this misleading "convenience fee" legal?
- Calling get_GeodesicArea from ogr2ogr
- How can I put node of a forest correctly?
- Is sudoku only one puzzle?
- How "the unity of opposites" represents non-duality?
- Drawing an arc on a rectangle
- How to make outhouses less icky?
- Explaining Arithmetic Progression
- How do closed cycle liquid engines actually work?
- Seven different digits are placed in a row. The products of the first 3, middle 3 and last 3 are all equal. What is the middle digit?
- Retroactively specifying `-only` or `-or-later` for GPLv2 in an adopted project
- Does the overall mAh of the battery add up when batteries are parallel?
- "Knocking it out of the park" sports metaphor American English vs British English?
- Are there any virtues in virtue ethics that cannot be plausibly grounded in more fundamental utilitarian principles?
- block-structure matrix
- Why name the staves in LilyPond's "published" "Solo piano" template?
- The Reforger NPC is stuck underground. How do I get him to move?
- What do all branches of Mathematics have in common to be considered "Mathematics", or parts of the same field?
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Bitwise and shift operators (C# reference)
- 9 contributors
The bitwise and shift operators include unary bitwise complement, binary left and right shift, unsigned right shift, and the binary logical AND, OR, and exclusive OR operators. These operands take operands of the integral numeric types or the char type.
- Unary ~ (bitwise complement) operator
- Binary << (left shift) , >> (right shift) , and >>> (unsigned right shift) operators
- Binary & (logical AND) , | (logical OR) , and ^ (logical exclusive OR) operators
Those operators are defined for the int , uint , long , and ulong types. When both operands are of other integral types ( sbyte , byte , short , ushort , or char ), their values are converted to the int type, which is also the result type of an operation. When operands are of different integral types, their values are converted to the closest containing integral type. For more information, see the Numeric promotions section of the C# language specification . The compound operators (such as >>= ) don't convert their arguments to int or have the result type as int .
The & , | , and ^ operators are also defined for operands of the bool type. For more information, see Boolean logical operators .
Bitwise and shift operations never cause overflow and produce the same results in checked and unchecked contexts.
- Bitwise complement operator ~
The ~ operator produces a bitwise complement of its operand by reversing each bit:
You can also use the ~ symbol to declare finalizers. For more information, see Finalizers .
Left-shift operator <<
The << operator shifts its left-hand operand left by the number of bits defined by its right-hand operand. For information about how the right-hand operand defines the shift count, see the Shift count of the shift operators section.
The left-shift operation discards the high-order bits that are outside the range of the result type and sets the low-order empty bit positions to zero, as the following example shows:
Because the shift operators are defined only for the int , uint , long , and ulong types, the result of an operation always contains at least 32 bits. If the left-hand operand is of another integral type ( sbyte , byte , short , ushort , or char ), its value is converted to the int type, as the following example shows:
Right-shift operator >>
The >> operator shifts its left-hand operand right by the number of bits defined by its right-hand operand. For information about how the right-hand operand defines the shift count, see the Shift count of the shift operators section.
The right-shift operation discards the low-order bits, as the following example shows:
The high-order empty bit positions are set based on the type of the left-hand operand as follows:
If the left-hand operand is of type int or long , the right-shift operator performs an arithmetic shift: the value of the most significant bit (the sign bit) of the left-hand operand is propagated to the high-order empty bit positions. That is, the high-order empty bit positions are set to zero if the left-hand operand is non-negative and set to one if it's negative.
If the left-hand operand is of type uint or ulong , the right-shift operator performs a logical shift: the high-order empty bit positions are always set to zero.
Use the unsigned right-shift operator to perform a logical shift on operands of signed integer types. This is preferred to casting a left-hand operand to an unsigned type and then casting the result of a shift operation back to a signed type.
Unsigned right-shift operator >>>
Available in C# 11 and later, the >>> operator shifts its left-hand operand right by the number of bits defined by its right-hand operand. For information about how the right-hand operand defines the shift count, see the Shift count of the shift operators section.
The >>> operator always performs a logical shift. That is, the high-order empty bit positions are always set to zero, regardless of the type of the left-hand operand. The >> operator performs an arithmetic shift (that is, the value of the most significant bit is propagated to the high-order empty bit positions) if the left-hand operand is of a signed type. The following example demonstrates the difference between >> and >>> operators for a negative left-hand operand:
- Logical AND operator &
The & operator computes the bitwise logical AND of its integral operands:
For bool operands, the & operator computes the logical AND of its operands. The unary & operator is the address-of operator .
- Logical exclusive OR operator ^
The ^ operator computes the bitwise logical exclusive OR, also known as the bitwise logical XOR, of its integral operands:
For bool operands, the ^ operator computes the logical exclusive OR of its operands.
- Logical OR operator |
The | operator computes the bitwise logical OR of its integral operands:
For bool operands, the | operator computes the logical OR of its operands.
- Compound assignment
For a binary operator op , a compound assignment expression of the form
is equivalent to
except that x is only evaluated once.
The following example demonstrates the usage of compound assignment with bitwise and shift operators:
Because of numeric promotions , the result of the op operation might be not implicitly convertible to the type T of x . In such a case, if op is a predefined operator and the result of the operation is explicitly convertible to the type T of x , a compound assignment expression of the form x op= y is equivalent to x = (T)(x op y) , except that x is only evaluated once. The following example demonstrates that behavior:
Operator precedence
The following list orders bitwise and shift operators starting from the highest precedence to the lowest:
- Shift operators << , >> , and >>>
Use parentheses, () , to change the order of evaluation imposed by operator precedence:
For the complete list of C# operators ordered by precedence level, see the Operator precedence section of the C# operators article.
Shift count of the shift operators
For the built-in shift operators << , >> , and >>> , the type of the right-hand operand must be int or a type that has a predefined implicit numeric conversion to int .
For the x << count , x >> count , and x >>> count expressions, the actual shift count depends on the type of x as follows:
If the type of x is int or uint , the shift count is defined by the low-order five bits of the right-hand operand. That is, the shift count is computed from count & 0x1F (or count & 0b_1_1111 ).
If the type of x is long or ulong , the shift count is defined by the low-order six bits of the right-hand operand. That is, the shift count is computed from count & 0x3F (or count & 0b_11_1111 ).
The following example demonstrates that behavior:
As the preceding example shows, the result of a shift operation can be non-zero even if the value of the right-hand operand is greater than the number of bits in the left-hand operand.
Enumeration logical operators
The ~ , & , | , and ^ operators are also supported by any enumeration type. For operands of the same enumeration type, a logical operation is performed on the corresponding values of the underlying integral type. For example, for any x and y of an enumeration type T with an underlying type U , the x & y expression produces the same result as the (T)((U)x & (U)y) expression.
You typically use bitwise logical operators with an enumeration type that is defined with the Flags attribute. For more information, see the Enumeration types as bit flags section of the Enumeration types article.
Operator overloadability
A user-defined type can overload the ~ , << , >> , >>> , & , | , and ^ operators. When a binary operator is overloaded, the corresponding compound assignment operator is also implicitly overloaded. A user-defined type can't explicitly overload a compound assignment operator.
If a user-defined type T overloads the << , >> , or >>> operator, the type of the left-hand operand must be T . In C# 10 and earlier, the type of the right-hand operand must be int ; beginning with C# 11, the type of the right-hand operand of an overloaded shift operator can be any.
C# language specification
For more information, see the following sections of the C# language specification :
- Bitwise complement operator
- Shift operators
- Logical operators
- Numeric promotions
- C# 11 - Relaxed shift requirements
- C# 11 - Logical right-shift operator
- C# operators and expressions
- Boolean logical operators
Additional resources

COMMENTS
In this article. The assignment operator = assigns the value of its right-hand operand to a variable, a property, or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or ...
The using directive imports a namespace so that you don't have to explicitly use the namespace to prefix all the types within it. Your particular variant basically creates an alias for the EPiServer.Enterprise.Diff.TreeNode namespace so you can simply refer to it as DiffTreeNode beyond that point. In other words, after the directive:
In particular, the using statement ensures that a disposable instance is disposed even if an exception occurs within the block of the using statement. In the preceding example, an opened file is closed after all lines are processed. Use the await using statement to correctly use an IAsyncDisposable instance: C#. Copy.
How is the Dispose Method Automatically Called in C#? When we use the using statement in C# for creating any object, behind the scenes, the compiler will create a code block using try/finally to make sure Dispose method is also called even though an exception is thrown. This is because the finally block gives you the guarantee to be executed irrespective of whether the exception is thrown in ...
Assignment Operators. Assignment operators are used to assign values to variables. In the example below, we use the assignment operator ( =) to assign the value 10 to a variable called x: Example. int x = 10; Try it Yourself ». The addition assignment operator ( +=) adds a value to a variable: Example. int x = 10;
If the assignment fails then dog is null, which prevents the contents of the for loop from running, because it is immediately broken out of. If the assignment succeeds then the for loop runs through the iteration. At the end of the iteration, the dog variable is assigned a value of null, which breaks out of the for loop.
In c#, Assignment Operators are useful to assign a new value to the operand, and these operators will work with only one operand. For example, we can declare and assign a value to the variable using the assignment operator ( =) like as shown below. int a; a = 10; If you observe the above sample, we defined a variable called " a " and ...
Simple assignment operator, Assigns values from right side operands to left side operand. C = A + B assigns value of A + B into C. +=. Add AND assignment operator, It adds right operand to the left operand and assign the result to left operand. C += A is equivalent to C = C + A.
For example, consider the expression 2 + 3 = 5, here 2 and 3 are operands, and + and = are called operators. So, the Operators in C# are used to manipulate the variables and values in a program. int x = 10, y = 20; int result1 = x + y; //Operator Manipulating Variables, where x and y are variables and + is operator.
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Use the Nullable<T>.GetValueOrDefault() method if the value to be used when a nullable type value is null should be the default value of the underlying value type. You can use a throw expression as the right-hand operand of the ?? operator to make the argument-checking code more concise:
Assignment Operators. Conditional Operator. In C#, Operators can also categorized based upon Number of Operands : Unary Operator: Operator that takes one operand to perform the operation. Binary Operator: Operator that takes two operands to perform the operation. Ternary Operator: Operator that takes three operands to perform the operation.
It matters for two reasons. One is conventional, the other technical. The conventional reason is that _ conveys active disinterest in the returned value. Sure, you could write var dontcare instead, but that's just a different arbitrary value. But as you pointed out, you could also omit the assignment, so it's not just about choosing the ...
To perform delegate removal, use the -operator. For more information about delegate types, see Delegates. Addition assignment operator += An expression using the += operator, such as. x += y is equivalent to. x = x + y except that x is only evaluated once. The following example demonstrates the usage of the += operator:
If you're interested in having a "switch" expression, you can find the little fluent class that I wrote here in order to have code like this: a = Switch.On(b) .Case(c).Then(d) .Case(e).Then(f) .Default(g); You could go one step further and generalize this even more with function parameters to the Then methods.
See also. The conditional operator ?:, also known as the ternary conditional operator, evaluates a Boolean expression and returns the result of one of the two expressions, depending on whether the Boolean expression evaluates to true or false, as the following example shows: C#. Copy. Run.
For the complete list of C# operators ordered by precedence level, see the Operator precedence section of the C# operators article. Operator overloadability. A user-defined type can overload the !, &, |, and ^ operators. When a binary operator is overloaded, the corresponding compound assignment operator is also implicitly overloaded.
BtnClickHandler2. If you want specific info about += operator, MSDN says: The += operator is also used to specify a method that will be called in response to an event; such methods are called event handlers. The use of the += operator in this context is referred to as subscribing to an event. For more info look at:
Unsigned right-shift operator >>> Available in C# 11 and later, the >>> operator shifts its left-hand operand right by the number of bits defined by its right-hand operand. For information about how the right-hand operand defines the shift count, see the Shift count of the shift operators section.. The >>> operator always performs a logical shift. That is, the high-order empty bit positions ...