- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter

How to convert speech into text using JavaScript ?
In this article, we will learn to convert speech into text using HTML and JavaScript.
Approach: We added a content editable “div” by which we make any HTML element editable.
We use the SpeechRecognition object to convert the speech into text and then display the text on the screen.
We also added WebKit Speech Recognition to perform speech recognition in Google chrome and Apple safari.
InterimResults results should be returned true and the default value of this is false. So set interimResults= true
Use appendChild() method to append a node as the last child of a node.
Add eventListener, in this event listener, map() method is used to create a new array with the results of calling a function for every array element.
Note: This method does not change the original array.
Use join() method to return array as a string.
Final Code:
Output:
If the user tells “Hello World” after running the file, it shows the following on the screen.
Please Login to comment...
Similar reads.
- Technical Scripter
- Web Technologies
- JavaScript-Misc
- Technical Scripter 2020
- Best PS5 SSDs in 2024: Top Picks for Expanding Your Storage
- Best Nintendo Switch Controllers in 2024
- Xbox Game Pass Ultimate: Features, Benefits, and Pricing in 2024
- Xbox Game Pass vs. Xbox Game Pass Ultimate: Which is Right for You?
- Full Stack Developer Roadmap [2024 Updated]
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

DEV Community

Posted on Jan 2
Building a Real-time Speech-to-text Web App with Web Speech API
Happy New Year, everyone! In this short tutorial, we will build a simple yet useful real-time speech-to-text web app using the Web Speech API. Feature-wise, it will be straightforward: click a button to start recording, and your speech will be converted to text, displayed in real-time on the screen. We'll also play with voice commands; saying "stop recording" will halt the recording. Sounds fun? Okay, let's get into it. 😊
Web Speech API Overview
The Web Speech API is a browser technology that enables developers to integrate speech recognition and synthesis capabilities into web applications. It opens up possibilities for creating hands-free and voice-controlled features, enhancing accessibility and user experience.
Some use cases for the Web Speech API include voice commands, voice-driven interfaces, transcription services, and more.
Let's Get Started
Now, let's dive into building our real-time speech-to-text web app. I'm going to use vite.js to initiate the project, but feel free to use any build tool of your choice or none at all for this mini demo project.
- Create a new vite project:
- Choose "Vanilla" on the next screen and "JavaScript" on the following one. Use arrow keys on your keyboard to navigate up and down.
HTML Structure
CSS Styling
JavaScript Implementation
This simple web app utilizes the Web Speech API to convert spoken words into text in real-time. Users can start and stop recording with the provided buttons. Customize the design and functionalities further based on your project requirements.
Final demo: https://stt.nixx.dev
Feel free to explore the complete code on the GitHub repository .
Now, you have a basic understanding of how to create a real-time speech-to-text web app using the Web Speech API. Experiment with additional features and enhancements to make it even more versatile and user-friendly. 😊 🙏
Top comments (0)
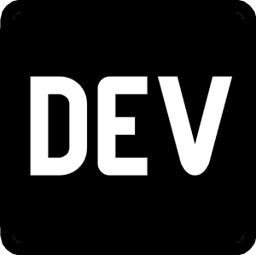
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
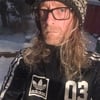
nginx: putting your site in ‘downtime’ for everyone except you
grant horwood - Sep 6

🚀 Appwrite: Revolutionizing Backend Development for Developers
Sachin Gadekar - Sep 10

How to Run FLUX1 for Free: A Step-by-Step Guide
Felippe Chemello - Sep 9

O que é uma Anticorruption Layer?
Pedro Augusto - Sep 11
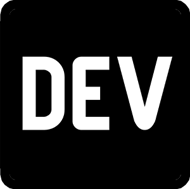
We're a place where coders share, stay up-to-date and grow their careers.
JavaScript Speech Recognition Example (Speech to Text)
With the Web Speech API, we can recognize speech using JavaScript . It is super easy to recognize speech in a browser using JavaScript and then getting the text from the speech to use as user input. We have already covered How to convert Text to Speech in Javascript .
But the support for this API is limited to the Chrome browser only . So if you are viewing this example in some other browser, the live example below might not work.

This tutorial will cover a basic example where we will cover speech to text. We will ask the user to speak something and we will use the SpeechRecognition object to convert the speech into text and then display the text on the screen.
The Web Speech API of Javascript can be used for multiple other use cases. We can provide a list of rules for words or sentences as grammar using the SpeechGrammarList object, which will be used to recognize and validate user input from speech.
For example, consider that you have a webpage on which you show a Quiz, with a question and 4 available options and the user has to select the correct option. In this, we can set the grammar for speech recognition with only the options for the question, hence whatever the user speaks, if it is not one of the 4 options, it will not be recognized.
We can use grammar, to define rules for speech recognition, configuring what our app understands and what it doesn't understand.
JavaScript Speech to Text
In the code example below, we will use the SpeechRecognition object. We haven't used too many properties and are relying on the default values. We have a simple HTML webpage in the example, where we have a button to initiate the speech recognition.
The main JavaScript code which is listening to what user speaks and then converting it to text is this:
In the above code, we have used:
recognition.start() method is used to start the speech recognition.
Once we begin speech recognition, the onstart event handler can be used to inform the user that speech recognition has started and they should speak into the mocrophone.
When the user is done speaking, the onresult event handler will have the result. The SpeechRecognitionEvent results property returns a SpeechRecognitionResultList object. The SpeechRecognitionResultList object contains SpeechRecognitionResult objects. It has a getter so it can be accessed like an array. The first [0] returns the SpeechRecognitionResult at the last position. Each SpeechRecognitionResult object contains SpeechRecognitionAlternative objects that contain individual results. These also have getters so they can be accessed like arrays. The second [0] returns the SpeechRecognitionAlternative at position 0 . We then return the transcript property of the SpeechRecognitionAlternative object.
Same is done for the confidence property to get the accuracy of the result as evaluated by the API.
We have many event handlers, to handle the events surrounding the speech recognition process. One such event is onspeechend , which we have used in our code to call the stop() method of the SpeechRecognition object to stop the recognition process.
Now let's see the running code:
When you will run the code, the browser will ask for permission to use your Microphone , so please click on Allow and then speak anything to see the script in action.
Conclusion:
So in this tutorial we learned how we can use Javascript to write our own small application for converting speech into text and then displaying the text output on screen. We also made the whole process more interactive by using the various event handlers available in the SpeechRecognition interface. In future I will try to cover some simple web application ideas using this feature of Javascript to help you usnderstand where we can use this feature.
If you face any issue running the above script, post in the comment section below. Remember, only Chrome browser supports it .
You may also like:
- JavaScript Window Object
- JavaScript Number Object
- JavaScript Functions
- JavaScript Document Object
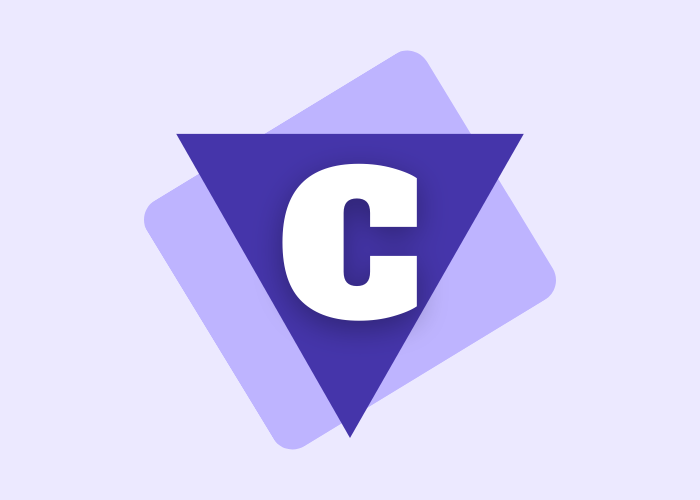
IF YOU LIKE IT, THEN SHARE IT
Related posts.
Converting from Speech to Text with JavaScript
In this tutorial we are going to experiment with the Web Speech API . It's a very powerful browser interface that allows you to record human speech and convert it into text. We will also use it to do the opposite - reading out strings in a human-like voice.
Let's jump right in!
To showcase the ability of the API we are going to build a simple voice-powered note app. It does 3 things:
- Takes notes by using voice-to-text or traditional keyboard input.
- Saves notes to localStorage.
- Shows all notes and gives the option to listen to them via Speech Synthesis.
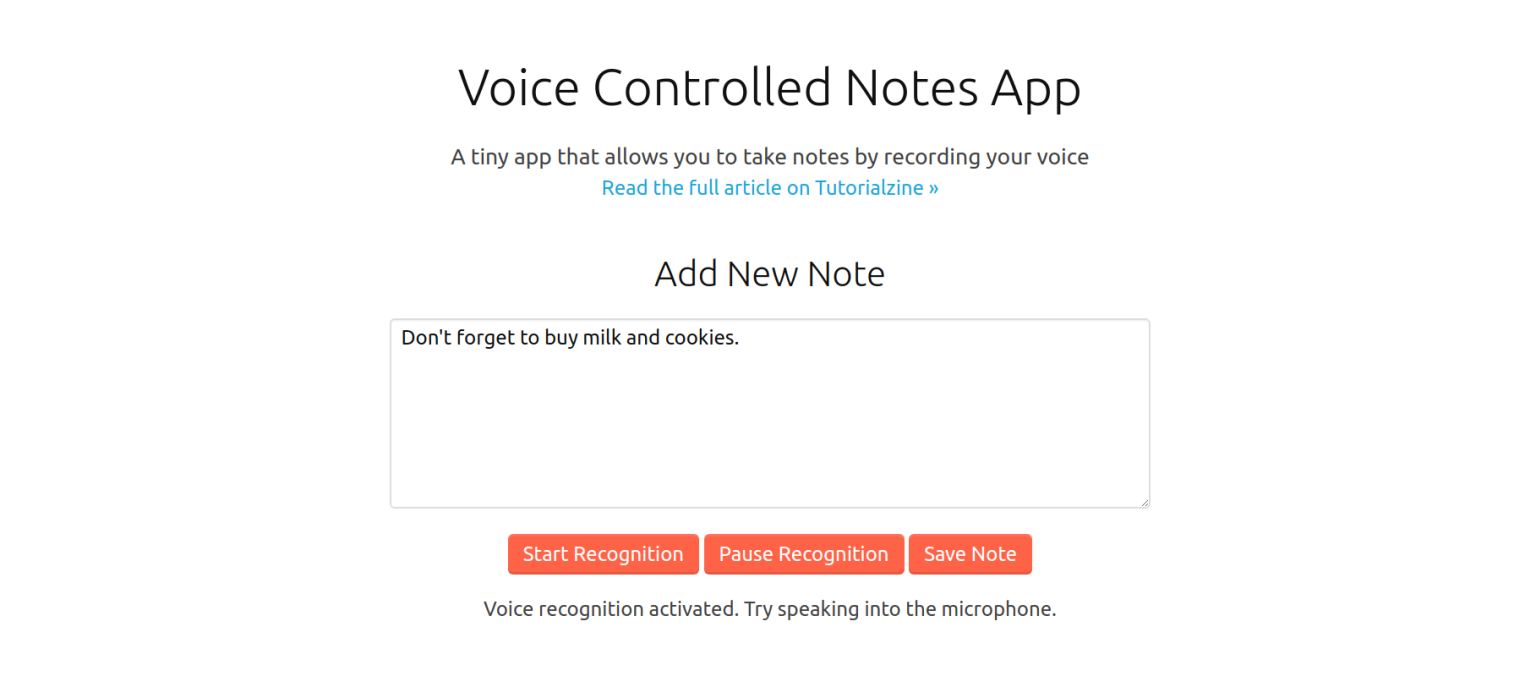
We won't be using any fancy dependencies, just good old jQuery for easier DOM operations and Shoelace for CSS styles. We are going to include them directly via CDN, no need to get NPM involved for such a tiny project.
The HTML and CSS are pretty standard so we are going to skip them and go straight to the JavaScript. To view the full source code go to the Download button near the top of the page.
Speech to Text
The Web Speech API is actually separated into two totally independent interfaces. We have SpeechRecognition for understanding human voice and turning it into text (Speech -> Text) and SpeechSynthesis for reading strings out loud in a computer generated voice (Text -> Speech). We'll start with the former.
The Speech Recognition API is surprisingly accurate for a free browser feature. It recognized correctly almost all of my speaking and knew which words go together to form phrases that make sense. It also allows you to dictate special characters like full stops, question marks, and new lines.
The first thing we need to do is check if the user has access to the API and show an appropriate error message. Unfortunately, the speech-to-text API is supported only in Chrome and Firefox (with a flag), so a lot of people will probably see that message.
The recognition variable will give us access to all the API's methods and properties. There are various options available but we will only set recognition.continuous to true. This will enable users to speak with longer pauses between words and phrases.
Before we can use the voice recognition, we also have to set up a couple of event handlers. Most of them simply listen for changes in the recognition status:
There is, however, a special onresult event that is very crucial. It is executed every time the user speaks a word or several words in quick succession, giving us access to a text transcription of what was said.
When we capture something with the onresult handler we save it in a global variable and display it in a textarea:
The above code is slightly simplified. There is a very weird bug on Android devices that causes everything to be repeated twice. There is no official solution yet but we managed to solve the problem without any obvious side effects. With that bug in mind the code looks like this:
Once we have everything set up we can start using the browser's voice recognition feature. To start it simply call the start() method:
This will prompt users to give permission. If such is granted the device's microphone will be activated.
Most APIs that require user permission don't work on non-secure hosts. Make sure you are serving your Web Speech apps over HTTPS.
The browser will listen for a while and every recognized phrase or word will be transcribed. The API will stop listening automatically after a couple seconds of silence or when manually stopped.
With this, the speech-to-text portion of our app is complete! Now, let's do the opposite!
Text to Speech
Speech Synthesys is actually very easy. The API is accessible through the speechSynthesis object and there are a couple of methods for playing, pausing and other audio related stuff. It also has a couple of cool options that change the pitch, rate, and even the voice of the reader.
All we will actually need for our demo is the speak() method. It expects one argument, an instance of the beautifully named SpeechSynthesisUtterance class.
Here is the entire code needed to read out a string.
When this function is called, a robot voice will read out the given string, doing it's best human impression.
In an era where voice assistants are more popular then ever, an API like this gives you a quick shortcut to building bots that understand and speak human language.
Adding voice control to your apps can also be a great form of accessibility enhancement. Users with visual impairment can benefit from both speech-to-text and text-to-speech user interfaces.
The speech synthesis and speech recognition APIs work pretty well and handle different languages and accents with ease. Sadly, they have limited browser support for now which narrows their usage in production. If you need a more reliable form of speech recognition, take a look at these third-party APIs:
- Google Cloud Speech API
- Bing Speech API
- CMUSphinx and it's JavaScript version Pocketsphinx (both open-source).
- API.AI - Free Google API powered by Machine Learning
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Related Articles
The easiest way to add validation to your forms, neon text effect with jquery & css, generating files with javascript, converting jquery code to a plugin, the best javascript and css libraries for 2017, quick tip: detecting your location with javascript, comments 18.
Excellent article and very easy to follow.
I got to thinking, why not add CKEditor to the textarea and did so after downloading demo. Everything looks good, even the recording works and notes can be saved. BUT, the spoken wrods are not appearing in the CKEditor area.
When you have time, could you possible cover getting your tutorial working with CKEditor? Be a really nice addition to have:)
Thanks for sharing your notes tutorial!
THIS IS MY CURRENT ISSUE RIGHT NOW. DID YOU SOLVED IT MAN ?
Great tutorial! Just wondering why doesn't the Start Recognition button work if I copy the code to a Codeanywhere project?
excellent article. i didn't have idea that such API exists in browser. tried example in chrome , worked fine. how this work for non-english speaking language?
Both text-to-speech and speech-to-text work pretty well with other languages.
For speech recognition you have to set the recognition.lang property .
With speech synthesis you can change the speaking voice. There is a large list of different languages to choose from - getVoices .
Sorry, it doesn't work.
It's problem with your secure connection. Check Is it https or not ?
This is working excellent on my local server i have implemented this and uploaded it on hosting server when i start the recognition the page says , this page is always blocked from using the microphone . Can you help me ?
i am working on speech recognition for Android applications. please suggest me can i use it in android and how can i get jar file for artyom.js?
Nice tutorial!
Is there a way to change language for the recognizer?
previously I had one already developed, but this one started to give me problems in the activation of the microphone, so I've been looking for solutions, I see that this example works very well, but when downloading the code and even make a copy and use the current one, it does not work for me, I put it on my server and nothing, so I tried to put it in a hosting to see if the server was the problem, and in the same way it does not connect, the microphone is not activated, which is what I will be missing for that this example works well, since I can not make functional the activation of the microphone, unless it enters by localhost, there is its that allows me to activate the microphone.
Firefox (with a flag) what about flag ?
It's working perfectly fine on Chrome... but, what changes we need to do so that it will work on all browsers..
Excellent articles and easy to understand and also easy to implement
why doesn't the Start Recognition button work if I copy the code in visual studio code ?
this is great 💥💥💥
great tutorial and library thank you so much for it, but actually i have problem when recognizing languages other than English have you made this library to work with other languages. my case: i am trying to recognize Arabic and turn an Arabic speech to text but the Arabic written in English letters and that is not correct
Bootstrap Studio Exclusive
This snippet is available only in Bootstrap Studio, the most powerful drag and drop website builder ever.
Oops! Something went wrong :( Try again...
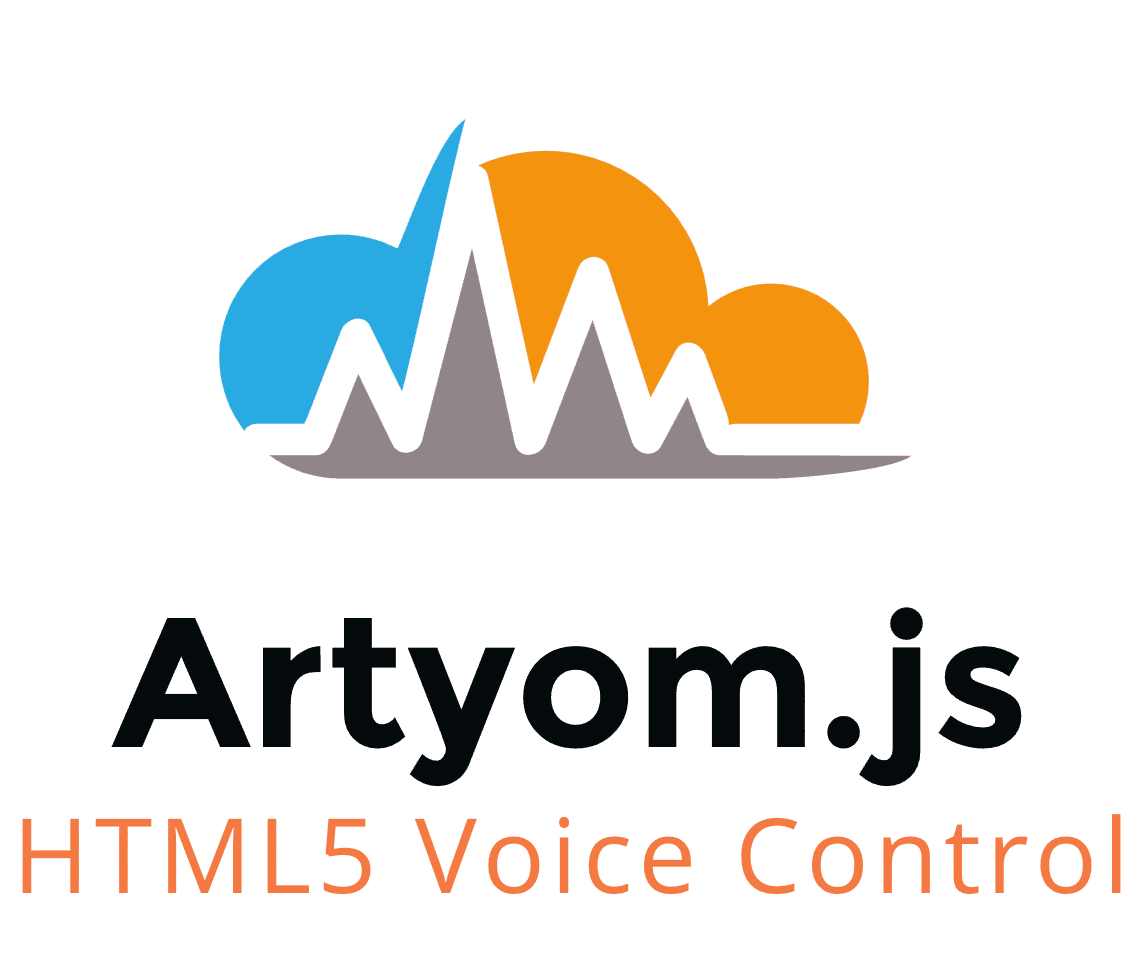
Voice commands and speech synthesis made easy
Artyom.js is an useful wrapper of the speechSynthesis and webkitSpeechRecognition APIs.
Besides, artyom.js also lets you to add voice commands to your website easily, build your own Google Now, Siri or Cortana !
Download .js
Get on bower, installation.
If you don't use any module bundler like browserify, require etc, just include the artyom window script in the head tag of your document and you are ready to go !
The Artyom class would be now available and you can instantiate it:
Note You need to load artyom.js in the head tag to preload the voices in case you want to use the speechSynthesis API. otherwise you can still load it in the end of the body tag.
About Artyom in this Browser
Loading info ....
According to your browser, speech synthesis and speech recognition may be available or not separately, use artyom.speechSupported and artyom.recognizingSupported methods to know it.
These are the available voices of artyom in this browser. See the initialization codes in the initialization area or read the docs.
Our Code Editor
Give artyom some orders in this website Since you're in this website artyom has been enabled. Try using any of the demo commands in the following list to test it !
Trigger command with | Description | Smart |
---|
Voice commands
Before the initialization, we need to add some commands for being processed. Use the artyom.addCommands(commands) method to add commands.
A command is a literal object with some properties. There are 2 types of commands normal and smarts .
A smart command allow you to retrieve a value from a spoken string as a wildcard. Every command can be triggered for any of the identifiers given in the indexes array.
Pro tip You can add commands dinamically while artyom is active. The commands are stored in an array so you can add them whenever you want and they'll be processed.
Start artyom
Now that artyom has commands, these can be processed. Artyom can work in continuous and uncontinuous mode.
Remember that artyom provides you the possibility to process the commands with a server language instead of javascript, you can enable the remote mode of artyom and use the artyom.remoteProcessorService method.
Note You'll need an SSL certificate in your website (https connection) in order to use the continuous mode, otherwise you'll be prompted for the permission to access the microphone everytime the recognition ends.
Pro tip Set always the debug property to true if you're working with artyom locally , you'll find convenient, valuable messages and information in the browser console.
Speech text
Use artyom.say to speak text. The language is retrieven at the initialization from the lang property.
Note Artyom removes the limitation of the traditional API ( about 150 characters max. Read more about this issue here ). With artyom you can read very extense text chunks without being blocked and the onEnd and onStart callbacks will be respected.
Pro tip Split the text by yourself in the way you want and execute and use artyom.say many times to decrease the probability of limitation of characters in the spoken text.
Test it by yourself paste all the text you want in the following textarea and click on speak to hear it !
Speech to text
Convert what you say into text easily with the dictation object.
Note You'll need to stop artyom before start a new dictation using artyom.fatality as 2 instances of webkitSpeechRecognition cannot run at time.
Simulate instructions without say a word
You can simulate a command without use the microphone using artyom.simulateInstruction("command identifier") for test purposes (or you don't have any microphone for test).
Try simulating any of the commands of this document like "hello","go to github" etc.
Get spoken text while artyom is active
If you want to show the user the recognized text while artyom is active, you can redirect the output of the speech recognition of artyom using artyom.redirectRecognizedTextOutput .
All that you say on this website will be shown in the following box:
Pause and resume commands recognition
You can pause the commands recognition, not the original speechRecognition. The text recognition will continue but the commands execution will be paused using the artyom.dontObey method.
To resume the command recognition use the artyom.obey . Alternatively, use the obeyKeyword property to enable with the voice at the initialization.
Useful keywords
Use the executionKeyword at the initialization to execute immediately a command though you are still talking. Use the obeyKeyword to resume the commands recognition if you use the pause method ( artyom.dontObey ). If you say this keyword while artyom is paused, artyom will be resumed and it will continue processing commands automatically.
Trending tops in Our Code World
Top 7 : best free web development ide for javascript, html and css.
See the review from 7 of the best free IDE (and code editors) for web proyects development in Our Code World.
Top 5 : Best jQuery scheduler and events calendar for web applications
See the review from 5 of the best dynamics scheduler and events calendar for Web applications with Javascript and jQuery in Our Code World
Top 20: Best free bootstrap admin templates
See the collection from 20 of the most imponent Admin templates built in bootstrap for free in Our Code World.
Thanks for read everything !
Support the project, did you like artyom.
If you did, please consider in give a star on the github repository and share this project with your developer friends !
We are already persons supporting artyom.js
I'm here to help you
Issues and troubleshooting.
If you need help while you're trying to implement artyom and something is not working, or you have suggestions please report a ticket in the issues are on github and i'll try to help you ASAP.
talkify-tts
- 0 Dependencies
- 1 Dependents
- 47 Versions
A javascript text to speech (TTS) library. Originally from and used by https://talkify.net .
Give a voice to your website in a matter of minutes. Talkify library provides you with high quality text to speech (TTS) voices in many languages.
To use our backend services (our hosted voices) you will require an api-key. Visit our portal ( https://manage.talkify.net ) to create your own API-key, Talkify offers 1000 free requests per month.
Dependencies
Configuration.
- Form reader
Text selection reader
Installation.
Font Awesome 5+ (Used in Talkify Control Center)
Quick demos
- Web Reader http://jsfiddle.net/5atrbjc6/
- Form Reader http://jsfiddle.net/dx53bg6k/2/
- Text selection Reader http://jsfiddle.net/t5dbcL64/
- Enhanced text visibility http://jsfiddle.net/pwbqkzxj/2/
Include the scripts and stylesheets
Minified version, non-minified version, stylesheets.
You find our stylesheets under /styles folder. Include the stylesheets that you need (i.e. all under /modern-control-center for our "modern" UI).
Play all, top to bottom
Play simple text.
High qualiy voices ( https://manage.talkify.net/docs#voices )
Supported languages:
Text highligting for easy read along
Control pitch, pauses between words, volume, speech rate, phonation and much more
Download as mp3
Playback of entire website or paragraph/s of your choice
Fully integrated UI options
Read web forms aloud
Listen to selected text
Enhanced visibility features
When useSSML is active, Talkify will translate the following markup into SSML. This has the potential of creating a smoother voice experience.
HTML tags | SSML |
---|---|
h1 - h3 | emphasis strong |
b | emphasis strong |
strong | emphasis strong |
i | emphasis reduced |
em | emphasis strong |
br | break-strength strong |
Declarative settings
These settings are only supported by the TtsPlayer for now.
Talkify supports declarative settings. These settings will override general settings. The following attributes can be added to any element that Talkify is connected to. When these attributes are present, Talkify will use them as playback settings.
data-attribute | Accepted values | Example | Remarks |
---|---|---|---|
data-talkify-wordbreakms | [0, 10000] | data-talkify-wordbreakms="100" | |
data-talkify-pitch | [-5, 5] | data-talkify-pitch="-2" | |
data-talkify-rate | [-10, 10] | data-talkify-rate="-2" | |
data-talkify-voice | Any authorized voice | data-talkify-voice="David" | |
data-talkify-phonation | "soft", "normal" or "" | data-talkify-phonation="soft" | |
data-talkify-whisper | "true" or "false" | data-talkify-whisper="true" | |
data-talkify-whisper | "true" or "false" | data-talkify-whisper="true" | |
data-talkify-read-as-lowercase | "true" | data-talkify-read-as-lowercase="true" | Some voices spell out capital letters, which might be unwanted, this setting will read the content of the element as lower case |
WebReader demo
Talkify lives in its own namespace - talkify. Hence, everything below is scoped to that namespace (i.e. talkify.playlist, etc).
Auto scroll
Talkify provides an opt in auto scroll to the item to be played.
Activate the feature by calling talkify.autoScroll.activate()
| Method | | activate |
Playlist fluent builder
Playlist builder is Talkifys way to instantiate your playlist. It comes with a fluent API.
Entry point: talkify.playlist()
Method | Parameters | Default | Description | Mandatory |
---|---|---|---|---|
begin | Entry point. Call this to start building your playlist | Yes | ||
usingPlayer | TtsPlayer/Html5Player | Specify which player to be used. | Yes | |
withTextInteraction | Enables you to click on paragraphs (and other text) to play | No | ||
withElements | DOM elements | Specifies with elements to play. If omitted, Talkify will crawl the page and select for you | No | |
excludeElements | Array of DOM-elements | [] | For example: document.querySelectorAll("button") | No |
withTables | Table configuration, array of objects* | Reads tables in a more intuitive way. The relevant header is repeated before each cell | No | |
withRootSelector | string | 'body' | Sets the scope from where Talkify will start to crawl the page for text to play | No |
subscribeTo | Json object | Event subscriptions | No | |
build | Finalizes and creates the playlist instance | Yes |
*withTables parameter is an array of objects with the following properties:
- table (DOM-query selector or actual DOM-elements)
- headerCells (Optional. DOM-query selector or actual DOM-elements. Defaults to "th")
- bodyCells (Optional. DOM-query selector or actual DOM-elements. Defaults to "td")
withTables works with any standard HTML-table and other non-standard tabular content (for example bootstrap grid system). For non standard tabular content, please use the optional parameters to tell Talkify which elements are header cells and which are body cells.
This is the instance built from the playliste above.
Method | Parameters | Default | Description |
---|---|---|---|
getQueue | Returns the playlist queue | ||
play | Begins playback of playlist | ||
pause | Pauses playlist | ||
replayCurrent | Replays the current item in the playlist | ||
insert | DOM element | Inserts new html elements to play. Useful for elements that Talkify were unable to locate. Elements will be inserted in correct order with respect to the page. | |
isPlaying | True if any item is currently in a playing state | ||
setPlayer | TtsPlayer/Html5Player | Sets the player that the playlist is using | |
enableTextInteraction | Enables click to play on HTML elements | ||
disableTextInteraction | Disables click to play on HTML elements | ||
dispose | Clean up |
Playlist Events
Event |
---|
onEnded |
onVoiceCommandListeningStarted |
onVoiceCommandListeningEnded |
Player (valid for all players)
Method | Parameters | Default | Description |
---|---|---|---|
enableTextHighlighting | Tells the player to use text highlighting. For Html5Player this only works on localVoice. | ||
disableTextHighlighting | Turns off text highlighting. | ||
subscribeTo | Json object | Event listeners | |
playText | string | Plays a text | |
paused | True if paused | ||
isPlaying | True if playing | ||
play | Play | ||
pause | Pause | ||
forceVoice | object | For Talkify hosted voices, this is a JSON object with a name property. The value of name should be the name of a voice from /api/speech/v1/voices. For browser voices, this is the actual voice from window.speechSynthesis.getVoices() | |
enableEnhancedTextVisibility | Enables enhanced text visibility. Subtitle-bar, with a larger font-size, is added to the bottom of the screen. | ||
disableEnhancedTextVisibility | Disables enhanced text visibility |
Html5Player only
Entry point: talkify.Html5Player().
Method | Parameters | Default | Description |
---|---|---|---|
forceLanguage | string | Force the usage of a specific language. Use standard cultures like se-SE for Swedish and so on. Talkify will select a voice that matches the culture. | |
setRate | double | 1 | [0.0, 2.0] Playback rate. |
setVolume | double | 1 | [0.0 - 1.0 ] |
usePitch | double | 1 | [0.0, 2.0] Adjusts the pitch of the voice. |
Talkify hosted only
Entry point: talkify.TtsPlayer(options?).
constructor parameter "options" is optional. Example { controlcenter: { container: document.querySelector('p.selector') , name: 'modern' }}
Method | Parameters | Default | Description |
---|---|---|---|
setRate | int | 1 | Playback rate. A value between -5 and 5 |
whisper | Sets the player to whispering mode | ||
normalTone | Sets the player to normal mode (opposite of whispering) | ||
usePhonation | string | normal | Supports for two phonations. "soft" and "normal". Empty string translates to "normal". Case sensitive |
useWordBreak | int | 0 | [0-10000] Adds a break between each word. Any value above 0 adds to the voices standard break length. |
usePitch | int | 0 | [-10 - +10] Adjusts the pitch of the voice. |
useVolumeBaseline | double | 0 | [-10 - +10] Adjusts the volume baseline |
Player Events
Event |
---|
onBeforeItemPlaying |
onSentenceComplete |
onPause |
onPlay |
onResume |
onItemLoaded |
onTextHighligtChanged |
Example: talkify.formReader.addForm(document.getElementById("form-id"));
Method | Parameters | Default | Description |
---|---|---|---|
addForm | form element | None | Adds TTS functionality to the form. |
removeForm | form element | None | Unbinds all TTS functionality from the form |
This feature allows the user to select/mark text using the mouse and have that text read aloud.
Method | Parameters | Default | Description |
---|---|---|---|
activate | - | - | Call this method to actiate the feature |
deactivate | - | - | Call this method to deactivate the feature |
withTextHighlighting | - | - | Presets text highlighting to activated. Users can turn this off in the control center UI |
withEnhancedVisibility | - | - | Presets enhanced visibility to activated. Users can turn this off in the control center UI |
withVoice | voice object | { name: 'Zira' } | A voice object from our backend voice API or at the very least an object wih a name property including a valid voice name |
withButtonText | string | "Listen" | The text that appears on popover button |
excludeElements | Array of DOM-elements | [] | For example: document.querySelectorAll("button") |
React to events
TLDR; Example @ http://jsfiddle.net/andreas_hagsten/x6pve0jd/8/
Talkify provides two event models - PubSub and classic callbacks. The newest, and primary, model is the PubSub model. PubSub is a loosly coupled model which enables client applications to hook in to the Talkify pipeline. To subscribe to events you will need to pass a context key (used when unsubscribing) as well as the event type and the event handler function. The event type is a string containing topics. An event is normally divided into 4 topics - context, origin, type and action.
The Context topic
You would use this top level topic if you run multiple instances of Talkify. This allows you to hook into a specific Talkify instance. If you want to listen to all instances or only have one just specify "*". You will find the context ID in the property "correlationId" of your Player instance.
The Origin topic
Where the event originates from. For example "player" or "controlcenter". A common use case is to listen to player events which is done by specifying "player" in this topic section.
The type topic
Type of event. For example "tts" for TTS-based events.
The action topic
This is the topic that describes what action is taken. This can be "play", "loading", "pause" and so forth.
Putting all 4 topics together forms the event type to listen to. You can replace any part with the wildcard "*" which means that you listens to all events of the given topic.
A few examples can be seen below. A full list of events supported is listed Here .
PubSub events
Type | args (TBD) |
---|---|
{contextId}.player.tts.ratechanged | |
{contextId}.player.tts.seeked | |
{contextId}.player.tts.pause | |
{contextId}.player.tts.timeupdated | |
{contextId}.player.tts.play | |
{contextId}.player.tts.resume | |
{contextId}.player.tts.loading | |
{contextId}.player.tts.loaded | |
{contextId}.player.tts.ended | |
{contextId}.player.tts.voiceset | |
{contextId}.player.tts.texthighlight.enabled | |
{contextId}.player.tts.texthighlight.disabled | |
{contextId}.player.tts.prepareplay | |
{contextId}.player.tts.disposed | |
{contextId}.player.tts.error | |
{contextId}.player.tts.phonationchanged | |
{contextId}.player.tts.whisperchanged | |
{contextId}.player.tts.wordbreakchanged | |
{contextId}.player.tts.volumechanged | |
{contextId}.player.tts.pitchchanged | |
{contextId}.player.tts.created | |
{contextId}.player.tts.unplayable | |
{contextId}.player.tts.enhancedvisibilityset | |
{contextId}.player.tts.creating | |
- | - |
{contextId}.player.html5.ratechanged | |
{contextId}.player.html5.pause | |
{contextId}.player.html5.utterancecomplete | |
{contextId}.player.html5.ended | |
{contextId}.player.html5.loaded | |
{contextId}.player.html5.play | |
{contextId}.player.html5.timeupdated | |
{contextId}.player.html5.voiceset | |
{contextId}.player.html5.texthighlight.enabled | |
{contextId}.player.html5.texthighlight.disabled | |
{contextId}.player.html5.prepareplay | |
{contextId}.player.html5.created | |
{contextId}.player.html5.unplayable | |
{contextId}.player.html5.enhancedvisibilityset | |
{contextId}.player.html5.creating | |
- | - |
{contextId}.controlcenter.request.play | |
{contextId}.controlcenter.request.pause | |
{contextId}.controlcenter.request.rate | |
{contextId}.controlcenter.request.volume | |
{contextId}.controlcenter.request.pitch | |
{contextId}.controlcenter.request.wordbreak | |
{contextId}.controlcenter.request.phonation.normal | |
{contextId}.controlcenter.request.phonation.soft | |
{contextId}.controlcenter.request.phonation.whisper | |
{contextId}.controlcenter.request.texthighlightoggled | |
{contextId}.controlcenter.request.textinteractiontoggled | |
{contextId}.controlcenter.request.enhancedvisibility | |
{contextId}.controlcenter.attached | |
{contextId}.controlcenter.detached | |
- | - |
{contextId}.wordhighlighter.complete | |
- | - |
{contextId}.playlist.playing | |
{contextId}.playlist.loaded | |
{contextId}.playlist.textinteraction.enabled | |
{contextId}.playlist.textinteraction.disabled |
- text to speech
- speech synthesis
Package Sidebar
npm i talkify-tts
Git github.com/Hagsten/Talkify
github.com/Hagsten/Talkify#readme
Downloads Weekly Downloads
Unpacked size, total files, last publish, collaborators.
Artisans Web
How To Guides & Programming Tutorials
How to Convert Live Speech to Text using JavaScript
Recently one of the readers asked about converting live speech to text. The topic sounds interesting to me. Though I wrote about converting speech to text using Amazon Transcribe and Google Cloud Speech , these services require passing audio files. These audio files are then converted into text.
But here the question is for live speech to text. So I decided to explore the solution and came across the Web Speech API . It provides 2 functionality – speech recognition, and speech synthesis. The speech recognition is used to get the text from the speech.
Speech recognition receives speech from your device’s microphone. The word or phrase is checked by a speech recognition service and then returned as a text string.
In this tutorial, we’ll convert live speech to text using Web Speech API and additionally create a PDF of this speech.
Note that Web Speech API is currently supported on a limited browser. You can use this service on the latest version of Chrome or Safari.
Getting Started
To see the flow in action, I’ll create the HTML with a few elements. We’ll have 2 buttons – Start and Stop to initiate and end speech recognition. When you click on the Start button, it first asks for permission to use the microphone. Once you give the permission, you can start speaking to your microphone. The words will start printing in HTML as you speak.
For ending the speech recognition, simply click the Stop button. As soon as you click it, a new button Save to PDF will appear. This button will convert your speech to PDF and send it to the browser.
Create the index.html file and add the following code to it.
Here, I am not adding any styling to the elements. The main purpose is to build the actual functionality. The design part will differ for each user.
I have included html2canvas and jspdf libraries via CDN into the HTML. These libraries generate the PDF out of HTML provided to it. It also has custom.js where we write the actual code for speech recognition and PDF generation.
In the HTML, I’ve added a div container with the class transcript . The text string of a speech will append inside this div container in the runtime.
Convert Live Speech to Text
At first, we must check browser compatibility for speech recognition and alert the user if it’s not supported.
Next, we have to create an object of the class SpeechRecognition . This class has few properties to interact with.
- continuous : If you want to continuously convert speech while speaking, set this property to true . It keeps speech recognition on until you explicitly end it.
- start : This property initiates the speech recognition service.
- stop : As the name suggests, it terminates the speech recognition process.
When you are talking to the microphone, Web Speech API starts recognizing words or phrases which need to catch and print on the page. For this, we have to use the onresult property of the SpeechRecognition class.
This code receives the text string runtime and keeps appending text to the specified div container. The process continues until you hit the Stop button.
Convert Speech to PDF
Once you are done with the process you might want to convert speech to PDF for offline use. To generate the PDF out of your text string, write the below code into the save_pdf() method.
It takes all content from the div having a class transcript and passes it to the jspdf library which then generates the PDF.
The final code of the custom.js file will be as follows.
You’re done with converting live speech to text using JavaScript. Give it a try and let me know your thoughts in the comment section below.
Related Articles
- Get YouTube Video List By Keywords Using YouTube Search API and JavaScript
- Validate Google reCAPTCHA using JavaScript
- How to Detect Browser in PHP and JavaScript
If you liked this article, then please subscribe to our YouTube Channel for video tutorials.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Popular Articles
- How to Integrate Google Sheets API with PHP
- How to Create a Meeting on Zoom using Zoom API and PHP
- Display Posts from Specific Category On A WordPress Page
- How to Install and Use CKEditor in Laravel
- How to Use DataTables in PHP
- How to Load WordPress Posts with AJAX
- Drag and Drop File Upload Using JavaScript and PHP
- How to Log Query in Laravel
- How to Read CSV and Excel File in PHP Using PhpSpreadsheet
- How to Install and Use TinyMCE Editor in Laravel
Email address:
Copyright © 2024 Artisans Web
Design by ThemesDNA.com
meSpeak.js (( • ))
Text-to-speech on the web.
Options: Amplitude: Pitch: Speed: Word gap: Variant: None f1 (female 1) f2 (female 2) f3 (female 3) f4 (female 4) f5 (female 5) m1 (male 1) m2 (male 2) m3 (male 3) m4 (male 4) m5 (male 5) m6 (male 6) m7 (male 7) croak klatt klatt2 klatt3 whisper whisperf (female)
Voice: ca - Catalan cs - Czech de - German el - Greek en - English en-n - English, regional en-rp - English, regional en-sc - English, Scottish en-us - English, US en-wm - English, regional eo - Esperanto es - Spanish es-la - Spanish, Latin America fi - Finnish fr - French hu - Hungarian it - Italian kn - Kannada la - Latin lv - Latvian nl - Dutch pl - Polish pt - Portuguese, Brazil pt-pt - Portuguese, European ro - Romanian sk - Slovak sv - Swedish tr - Turkish zh - Mandarin Chinese (Pinyin) zh-yue - Cantonese Chinese
First things first: Where can I download this? — See the download-link below.
meSpeak.js (modulary enhanced speak.js) is a 100% client-side JavaScript text-to-speech library based on the speak.js project, a port of the eSpeak speech synthesizer from C++ to JavaScript using Emscripten. meSpeak.js adds support for Webkit and Safari and introduces loadable voice modules. Also there is no more need for an embedding HTML-element. Separating the code of the library from voice definitions should help future optimizations of the core part of speak.js . All separated data has been compressed to base64-encoded strings from the original binary files to save some bandwidth (compared to JS-arrays of raw 8-bit data). All separated data has been compressed to base64-encoded strings from the original binary files to save some bandwidth (compared to JS-arrays of raw 8-bit data). Browser requirements: Firefox, Chrome/Opera, Webkit, and Safari (MSIE11 is expected to be compliant). meSpeak.js 2011-2020 by Norbert Landsteiner, mass:werk – media environments; https://www.masswerk.at/mespeak/
GNU General Public License The eSpeak text-to-speech project is licensed under version 3 of the GNU General Public License. Since meSpeak.js incorporates eSpeak, the same license (GPL v.3) applies.
Important Changes:
v 2.0 Major Upadate — Introducing a web worker for rendering the audio concurrently (outside the UI thread), reduced file size, basic audio filtering and stereo panning, and a new, simplified loading scheme for loading voice/language definitions. v 2.0.1 Added meSpeak.getAudioAnalyser() , because, why not? v 2.0.2 Disabled workers on mobile diveses. v 2.0.3 Changed implementation of meSpeak.getAudioAnalyser() . v 2.0.4 Added a simple mobile unlocker (initial touchstart event handler). (v. 2.0.5 Added the original eSpeak license statement.) v 2.0.6 Added a workaround an issue with some browsers after the 80 th call. v 2.0.7 Added audio unlocking for Safari desktop browsers.
Some real world examples (at masswerk.at): • Explore client-side speech I/O with E.L.I.Z.A. Talking • Celebrating meSpeak.js v.1.5: JavaScript Doing The JavaScript Rap (featuring MC meSpeak) (a heavy performance test) • Celebrating meSpeak.js v.2.0: MeSpeak.js Stereo Panning Demo (reading a dialog by distributed roles) • Audio Anaylser Demo , a simple oscilloscope display for meSpeak.js.
- MeSpeak now runs a worker in order to render any utterances, if available. (Otherwise, the core application is started in a single-threaded instance to maintain compatibility with older clients.) This means meSpeak.js will generally not block the UI thread and will also be precessing faster. Moreover, the filesize has been reduced (< 500K g-zipped) Please mind that workers are disabled for mobile devices. (Since there is no user interaction as the sound arrives from the worker on a postMessage event, the playback would be muted.)
- As a result, meSpeak.js now consists of two files, the fornt-end “ mespeak.js ” and the core application “ mespeak-core.js ”, which will be loaded automatically by the front-end. (You still have to include “ mespeak.js ” onyl, just as before.)
- A standard configuration is now included. Meaning, there is no need to call “ meSpeak.loadConfig() ” (which now does nothing) or checking meSpeak.isConfigLoaded() (which now returns always true .) However, there's now “ meSpeak.loadCustomConfig() ” to override the standard configuration.
- Voice files are now loaded relative to the script (instead of relative to the embedding page)! Also, you may now just specify a voice-ID and the respective JSON-file will be loaded from the directory “ voices ” in the same path as the application.
- In order to export a data-stream with the option “ rawdata ”, a callback has to be supplied. The stream will be returned as the third argumend (of success, id, stream) in the callback. “ meSpeak.speak() ” now always returns a 32-bit integer ID.
- There is now an additional option “ pan ” for stereo panning. Compare the Stereo Panning Demo .
- A new method “ meSpeak.setFilters() ” allows you to apply global audio filtering for prostprocessing. This may be any number of BiquadFilters or DynamicsCompressors as specified by the Web Audio API, which will be chained together and will feed into the global gain.
- The new method “ meSpeak.getAudioAnalyser() ” returns an Web Audio AnalyserNode for further processing (e.g., a wave display) of the signal played by meSpeak.js.
meSpeak.loadVoice('voices/en/en-us.json'); or just meSpeak.loadVoice('en/en-us'); meSpeak.speak('hello world'); meSpeak.speak('hello world', { option1: value1, option2: value2 .. }); meSpeak.speak('hello world', { option1: value1, option2: value2 .. }, myCallback); var id = meSpeak.speak('hello world'); meSpeak.stop(id); meSpeak.speak( text [, { option1: value1, option2: value2 .. } [, callback ]] ); text : The string of text to be spoken. The text may contain line-breaks ("\n") and special characters. Default text-encoding is UTF-8 (see the option "utf16" for other). options (eSpeak command-options): * amplitude : How loud the voice will be (default: 100) * pitch : The voice pitch (default: 50) * speed : The speed at which to talk (words per minute) (default: 175) * voice : Which voice to use (default: last voice loaded or defaultVoice, see below) * wordgap : Additional gap between words in 10 ms units (default: 0) * variant : One of the variants to be found in the eSpeak-directory "~/espeak-data/voices/!v" Variants add some effects to the normally plain voice, e.g. notably a female tone. Valid values are: "f1", "f2", "f3", "f4", "f5" for female voices "m1", "m2", "m3", "m4", "m5", "m6, "m7" for male voices "croak", "klatt", "klatt2", "klatt3", "whisper", "whisperf" for other effects. (Using eSpeak, these would be appended to the "-v" option by "+" and the value.) Note: Try "f2" or "f5" for a female voice. * linebreak : (Number) Line-break length, default value: 0. * capitals : (Number) Indicate words which begin with capital letters. 1: Use a click sound to indicate when a word starts with a capital letter, or double click if word is all capitals. 2: Speak the word "capital" before a word which begins with a capital letter. Other values: Increases the pitch for words which begin with a capital letter. The greater the value, the greater the increase in pitch. (eg.: 20) * punct : (Boolean or String) Speaks the names of punctuation characters when they are encountered in the text. If a string of characters is supplied, then only those listed punctuation characters are spoken, eg. { "punct": ".,;?" }. * nostop : (Boolean) Removes the end-of-sentence pause which normally occurs at the end of the text. * utf16 : (Boolean) Indicates that the input is UTF-16, default: UTF-8. * ssml : (Boolean) Indicates that the text contains SSML (Speech Synthesis Markup Language) tags or other XML tags. (A small set of HTML is supported too.) further options (meSpeak.js specific): * volume : Volume relative to the global volume (number, 0..1, default: 1) Note: the relative volume has no effect on the export using option 'rawdata'. * log : (Boolean) Logs the compiled eSpeak-command to the JS-console. * pan : (Number) Stereo panning, -1 >= pan <= 1 -1 represents the extreme left 1 represents the extreme right 0 center (no effect) This option is available only with clients supporting the Web Audio API. * rawdata : Do not play, return audio data (wav) in callback. (A callback, see below, has to be specified in order to retrieve the data stream.) The type of the returned data is derived from the value (case-insensitive) of 'rawdata': - ' base64 ': returns a base64-encoded string. - ' mime ': returns a base64-encoded data-url (including the MIME-header). (synonyms: 'data-url', 'data-uri', 'dataurl', 'datauri') - ' array ': returns a plain Array object with uint 8 bit data. - default (any other value): returns the generated wav-file as an ArrayBuffer (8-bit unsigned). Note: The value of 'rawdata' must evaluate to boolean 'true' in order to be recognized. callback : An optional callback function to be called after the sound output ended. function myCallback(success, id [, stream]) { ... } * success (Boolean): flag indicating the success of the operation * id (Number): 32-bit id, defaults to 0 * stream (*): data stream of the wav-file in the format specified by the "rawdata" option. Defaults to ArrayBuffer (uint8). If the resulting sound is stopped by meSpeak.stop() , the success-flag will be set to false. (A callbak may be also specified as a property of the options object. If both are present, the callback argument takes precedence.) Returns : * a 32bit integer ID greater than 0 (or 0 on failure). The ID may be used to stop this sound by calling meSpeak.stop( <id> ) . meSpeak.loadVoice('voices/fr.json', userCallback); meSpeak.loadVoice('en/en-us', userCallback); // userCallback is an optional callback-handler. The callback will receive two arguments: // * a boolean flag for success // * either the id of the voice, or a reason for errors ('network error', 'data error', 'file error') Note : Starting with meSpeak.js 2.0, voices are loaded relative to meSpeak.js . Also, if you just specify a voice-id, meSpeak.js will now try to load a respective voice from a directory "voices" in the same directory as the script. e.g., loadVoice('fr') will load ' /path/to/mespeak/ voices/fr.json', loadVoice('en/en-us') will load ' path/to/mespeak/ voices/en/en-us.json'. A newly loaded voice will always become the new default voice: meSpeak.loadVoice('fr'); alert( meSpeak.getDefaultVoice() ); // 'fr' meSpeak.setDefaultVoice('de'); Sets the default voice to the voice with the voice with the id specified. (Note: If not explicitly set the default voice is always the the last voice loaded.) if ( meSpeak.isVoiceLoaded('de') ) meSpeak.setDefaultVoice('de'); Check, if a voice has been successfully loaded. meSpeak.loadConfig() meSpeak.isConfigLoaded() Legacy methods. A standard configuration is now included in meSpeak.js. meSpeak.loadConfig() does nothing meSpeak.isConfigLoaded() returns always true However, you can still load a custom configuration using meSpeak.loadCustomConfig(url, callback) As with vocies, config-files will be loaded relative to the mespeak.js script. An optional callback will have two arguments, a boolean success flag and a message string reporting any reasons for failing the operation. A custom congiguration may include just some of the eSpeak config-files. Any files found, will overwrite the standard configurations. meSpeak.setVolume(0.5); meSpeak.setVolume( volume [, id-list] ); Sets a volume level (0 meSpeak.getVolume() ); // 0.5 meSpeak.getVolume( [id] ); Returns a volume level (0 meSpeak.canPlay(); // test for compatibility meSpeak.play( stream [, relativeVolume [, callback[, id[, pan]]]] ); Play (cached) audio streams (using any of the export formats, ArrayBuffer, array, base64, dta-URL) Arguments: stream : A stream in any of the formats returned by meSpeak.play() with the "rawdata"-option. volume : (optional) Volume relative to the global volume (number, 0..1, default: 1) callback : (optional) A callback function to be called after the sound output ended. The callback will be called with a single boolean argument indicating success. If the sound is stopped by meSpeak.stop() , the success-flag will be set to false. (See also: meSpeak.speak().) id : (optional, Number) An id to be used (default 0 => ignored.) meSpeak.play(myAudio, 1, null, mySoundId); meSpeak.stop(mySoundId); pan : (optional, Number) Stereo panning. (left) -1 >= pan <= 1 (right) Mind that this works only with clients supporting the Web Audio API. Returns : A 32bit integer ID greater than 0 (or 0 on failure). The ID may be used to stop this sound by calling meSpeak.stop( <id> ) . // exaple for caching and playing back audio streams var audiostreams = []; meSpeak.speak('hello world', { 'rawdata': true }, function(success, id, stream) { // data is ArrayBuffer of 8-bit uint audiostreams.push(stream); }); meSpeak.speak('hello again', { 'rawdata': 'array' }, function(success, id, stream) { // data is Array of 8-bit uint Numbers audiostreams.push(stream); }); meSpeak.speak('hello again', { 'rawdata': 'base64' }, function(success, id, stream) { // data is a string containing the base64-encoded wav-file audiostreams.push(stream); }); meSpeak.speak('hello yet again', { 'rawdata': 'data-url' }, function(success, id, stream) { // data is a data-URL with MIME-header "data:audio/x-wav;base64" audiostreams.push(stream); }); meSpeak.play(audiostreams[0]); // using global volume meSpeak.play(audiostreams[1], 0.75); // 75% of global volume meSpeak.play(audiostreams[2], 0, null, 0, -1); // play if from the left meSpeak.play(audiostreams[3], 0, 0, 0, 0.25); // play it from a querter to the right meSpeak.stop( [<id-list>] ); Stops the sound(s) specified by the id-list . If called without an argument, all sounds currently playing, processed, or queued are stopped. Any callback(s) associated to the sound(s) will return false as the success-flag. Arguments: id-list : Any number of IDs returned by a call to meSpeak.speak() or meSpeak.play() . Returns : The number (integer) of sounds actually stopped. meSpeak.setFilter(<options>[,<options>]); New in meSpeak 2.0: Set filters for audio playback (post processing). Supported are any of the BiquadFilters and DynamicsCompressors . You may add any number of filters, which will be chained together before feeding into the gloabel gain node. Options: type: (String) Filter type, case-insenstitive BiquadFilters: 'lowpass', 'highpass', 'bandpass', 'lowshelf', 'highshelf', 'peaking', 'notch', 'allpass' DynamicsCompressor: 'dynamicscompressor' or 'compressor' For BiquadFilters: frequency (Number) Q (Number) gain (Number) detune (Number) For DynamicsCompressors: threshold (Number) knee (Number) ratio (Number) reduction (Number) attack (Number) release (Number) // Example: meSpeak.setFilter( { type: 'highpass', frequency: 85 }, { type: 'compressor', threshold: -10, knee: 40, ratio: 5, attack: 0, release: 0.25 }, { type: 'bandpass', frequency: 500, Q: 0.125, detune: 10 } ); myAnalyserNode = meSpeak.getAudioAnalyser(); returns an Web Audio AnalyserNode for further processing (e.g., a wave display) of the signal played by meSpeak.js. The AnalyserNode mirrors the signal present in the first global audio processing stage (after individual volume/gain), but before filters. Compare the Audio Anaylser Demo . meSpeak.getRunMode(); Determine, if the client is running a concurrent worker or a single-threaded instance. Returns either the string "worker" or "instance" meSpeak.restartWithInstance(); For testing purposes only: Restart MeSpeak forcing it to use an instance instead of a worker. Returns: nothing / void.
Note on export formats , ArrayBuffer (typed array, defaul) vs. simple array: The ArrayBuffer (8-bit unsigned) provides a stream ready to be played by the Web Audio API (as a value for a BufferSourceNode), while the plain array (JavaScript Array object) may be best for export (e.g. sending the data to Flash via Falsh's ExternalInterface). The default raw format (ArrayBuffer) is the preferred format for caching streams to be played later by meSpeak by calling meSpeak.play() , since it provides the least overhead in processing.
Recommended File Layout
In order to ensure the functionality of meSpeak.js, the following layout is strongly encouraged:
mespeak/ mespeak.js # required mespeak-core.js # required voices/ # default location ca.json cs.json de.json ...
Mind that you just require thos vocie definitions which you are actually using.
meSpeak.speakMultipart() — concatenating multiple voices
Using meSpeak.speakMultipart() you may mix multiple parts into a single utterance.
See the Multipart-Example for a demo.
The general form of meSpeak.speakMultipart() is analogous to meSpeak.speak() , but with an array of objects (the parts to be spoken) as the first argument (rather than a single text):
meSpeak.speakMultipart( <parts-array> [, <options-object> [, <callback-function> ]] ) ; meSpeak.speakMultipart( [ { text: "text-1", <other options> ] }, { text: "text-2", <other options> ] }, ... { text: "text-n", <other options> ] }, ], { option1: value1, option2: value2 .. }, callback ) ;
Only the the first argument is mandatory, any further arguments are optional. The parts-array must contain a single element (of type object) at least. For any other options refer to meSpeak.speak() . Any options supplied as the second argument will be used as defaults for the individual parts. (Same options provided with the individual parts will override these defaults.) The method returns — like meSpeak.speak() — either an ID, or, if called with the "rawdata" option (in the general options / second argument), a stream-buffer representing the generated wav-file.
Note on iOS and Mobile Limitations
iOS (currently supported only using Safari) provides a single audio-slot, playing only one sound at a time. Thus, any concurrent calls to meSpeak.speak() or meSpeak.play() will stop any other sound playing. Further, iOS reserves volume control to the user exclusively. Any attempt to change the volume by a script will remain without effect. Please note that you still need a user-interaction at the very beginning of the chain of events in order to have a sound played by iOS.
Note on Options
The first set of options listed above corresponds directly to options of the espeak command. For details see the eSpeak command documentation . The meSpeak.js-options and their espeak-counterparts are ( mespeak.speak() accepts both sets, but prefers the long form):
amplitude | -a |
wordgap | -g |
pitch | -p |
speed | -s |
voice | -v |
variant | -v<voice>+<variant> |
utf16 | -b 4 (default: -b 1) |
linebreak | -l |
capitals | -k |
nostop | -z |
ssml | -m |
punct | --punct[="<characters>"] |
Voices Currently Available
- ca (Catalan)
- de (German)
- en/en (English)
- en/en-n (English, regional)
- en/en-rp (English, regional)
- en/en-sc (English, Scottish)
- en/en-us (English, US)
- en/en-wm (English, regional)
- eo (Esperanto)
- es (Spanish)
- es-la (Spanish, Latin America)
- fi (Finnish)
- fr (French)
- hu (Hungarian)
- it (Italian)
- kn (Kannada)
- lv (Latvian)
- pl (Polish)
- pt (Portuguese, Brazil)
- pt-pt (Portuguese, European)
- ro (Romanian)
- sk (Slovak)
- sv (Swedish)
- tr (Turkish)
- zh (Mandarin Chinese, Pinyin) *
- zh-yue (Cantonese Chinese, Provisional) **
JSON File Formats
1) Config-data: "mespeak_config.json": The config-file includes all data to configure the tone (e.g.: male or female) of the electronic voice.
{ "config": "<base64-encoded octet stream>", "phontab": "<base64-encoded octet stream>", "phonindex": "<base64-encoded octet stream>", "phondata": "<base64-encoded octet stream>", "intonations": "<base64-encoded octet stream>" }
Finally the JSON object may include an optional voice-object (see below), that will be set up together with the config-data:
{ ... "voice": { <voice-data> } }
2) Voice-data: "voice.json": A voice-file includes the ids of the voice and the dictionary used by this voice, and the binary data of theses two files.
{ "voice_id": "<voice-identifier>", "dict_id": "<dict-identifier>", "dict": "<base64-encoded octet stream>", "voice": "<base64-encoded octet stream>" }
Alternatively the value of "voice" may be a text-string, if an additional property "voice_encoding": "text" is provided. This shold allow for quick changes and testing:
{ "voice_id": "<voice-identifier>", "dict_id": "<dict-identifier>", "dict": "<base64-encoded octet stream>", "voice": "<text-string>", "voice_encoding": "text" }
Both config-data and voice-data may be loaded and switched on the fly to (re-)configure meSpeak.js.
Extendet Voice Format, Mbrola Voices
In order to support Mbrola voices and other voices requiring a more flexible layout and/or additional data, there is also an extended voice format :
{ "voice_id": "<voice-identifier>", "voice": "<base64-encoded octet stream>" "files": [ { "path", "<rel-pathname>", "data", "<base64-encoded octet stream>" }, { "path", "<rel-pathname>", "data", "<text-string>", "encoding": "text" }, ... ] }
or (using a text-encoded voice-definition):
{ "voice_id": "<voice-identifier>", "voice": "<text-string>", "voice_encoding": "text" "files": [ { "path", "<rel-pathname>", "data", "<base64-encoded octet stream>" }, { "path", "<rel-pathname>", "data", "<text-string>", "encoding": "text" }, ... ] }
Only a valid voice-definition is required and optionally an array "files" which may be empty or contain any number of objects, containing a property "path" (relative file-path from the espeak-data-directory) and a property "data" , containing the file (either as base64-encoded data or as plain text, if there is also an optional property "encoding": "text" ).
In order to facilitate the use of Mbrola voices, for any "voice_id" beginning with "mb/mb-" only the part following the initial "mb/" will be used as the internal identifyer for the meSpeak.speak() method. (So any given voice_id "mb/mb-en1" will be translated to a voice "mb-en1" automatically. This applies to the speak-command only.)
Please don't ask for support on Mbrola voices (I don't have the faintest idea). Please refer to Mbrola section of the eSpeak documentation for a guide to setting up the required files locally. It should be possible to load these into meSpeak.js using the "extended voice format", since you may put any additional payload into the files-array. Please mind that you will still require a text-to-phoneme translator as stated in the eSpeak documentation (this is out of the scope of meSpeak.js).
Deferred Calls
In case that speak() is called before any voice data has been loaded, the call will be deferred and executed after set up. See this page for an example. You may reset the queue manually by calling
meSpeak.resetQueue();
Amplitude and Volume
There are now two separate parameters or options to control the volume of the spoken text: amplitude and volume. While amplitude affects the generation of the sound stream by the TTS-algorithm, volume controls the playback volume of the browser. By the use of volume you can cache a generated stream and still provide an individual volume level at playback time. Please note that there is a global volume (controlled by setVolume() ) and an individual volume level relative to the global one. Both default to 1 (max volume).
Notes on Chinese Languages and Voices
Please note that the Chinese voices do only support Pinyin input (phonetic transcript like " zhong1guo2 " for 中 + 国, China) for "zh" and simple one-to-one translation from single Simplified Chinese characters or Jyutping romanised text for "zh-yue".
The eSpeak documentation provides the following notes:
*) zh (Mandarin Chinese) : This speaks Pinyin text and Chinese characters. There is only a simple one-to-one translation of Chinese characters to a single Pinyin pronunciation. There is no attempt yet at recognising different pronunciations of Chinese characters in context, or of recognising sequences of characters as "words". The eSpeak installation includes a basic set of Chinese characters. More are available in an additional data file for Mandarin Chinese at: http://espeak.sourceforge.net/data/.
**) zh-yue (Cantonese Chinese, Provisional) : Just a naive simple one-to-one translation from single Simplified Chinese characters to phonetic equivalents in Cantonese. There is limited attempt at disambiguation, grouping characters into words, or adjusting tones according to their surrounding syllables. This voice needs Chinese character to phonetic translation data, which is available as a separate download for Cantonese at: http://espeak.sourceforge.net/data/. The voice can also read Jyutping romanised text.
For a simple zh-to-Pinyin translation in JavaScript see: https://www.masswerk.at/mespeak/zh-pinyin-translator.zip
Flash-Fallback for Wave Files
(m)eSpeak produces internally wav-files, which are then played. Internet Explorer 10 supports typed arrays (which are required for the binary logic), but does not provide native playback of wav-files. To provide compatibility for this browser, you could try the experimental meSpeak Flash Fallback .
Download (all code under GPL): mespeak.zip (v.2.0.7, last update: 2020-04-23)
The last version of the old API, v.1.9.7.1 may be downloaded here: mespeak_1-9-7-1.zip
Version History
/* Cross-Browser Web Audio API Playback With Chrome And Callbacks */ // alias the Web Audio API AudioContext-object var aliasedAudioContext = window.AudioContext || window.webkitAudioContext; // ugly user-agent-string sniffing var isChrome = ((typeof navigator !== 'undefined') && navigator.userAgent && navigator.userAgent.indexOf('Chrome') !== -1); var chromeVersion = (isChrome)? parseInt( navigator.userAgent.replace(/^.*?\bChrome\/([0-9]+).*$/, '$1'), 10 ) : 0; function playSound(streamBuffer, callback) { // set up a BufferSource-node var audioContext = new aliasedAudioContext(); var source = audioContext.createBufferSource(); source.connect(audioContext.destination); // since the ended-event isn't generally implemented, // we need to use the decodeAudioData()-method in order // to extract the duration to be used as a timeout-delay audioContext.decodeAudioData(streamBuffer, function(audioData) { // detect any implementation of the ended-event // Chrome added support for the ended-event lately, // but it's unreliable (doesn't fire every time) // so let's exclude it. if (!isChrome && source.onended !== undefined) { // we could also use "source.addEventListener('ended', callback, false)" here source.onended = callback; } else { var duration = audioData.duration; // convert to msecs // use a default of 1 sec, if we lack a valid duration var delay = (duration)? Math.ceil(duration * 1000) : 1000; setTimeout(callback, delay); } // finally assign the buffer source.buffer = audioData; // start playback for Chrome >= 32 // please note that this would be without effect on iOS, since we're // inside an async callback and iOS requires direct user interaction if (chromeVersion >= 32) source.start(0); }, function(error) { /* decoding-error-callback */ }); // normal start of playback, this would be essentially autoplay // but is without any effect in Chrome 32 // let's exclude Chrome 32 and higher to avoid any double calls anyway if (!isChrome || chromeVersion < 32) { if (source.start) { source.start(0); } else { source.noteOn(0); } } }
About speak.js
speak.js is 100% clientside JavaScript. " speak.js " is a port of eSpeak , an open source speech synthesizer, which was compiled from C++ to JavaScript using Emscripten . The project page and source code for this demo can be found here . Note: There had been initially plans to merge this project with speak.js, but they somehow became stuck.
- Typed arrays . The eSpeak code is not portable to the extent that would be necessary to avoid using typed arrays. (It should however be possible to rewrite small bits of eSpeak to fix that.) Typed arrays are present in Firefox, Chrome, Webkit, and Safari, but not IE or Opera.
- Update : Opposed to the state of the original documentation, newer versions of Opera and IE both provide support for typed arrays.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Web Speech API
The Web Speech API enables you to incorporate voice data into web apps. The Web Speech API has two parts: SpeechSynthesis (Text-to-Speech), and SpeechRecognition (Asynchronous Speech Recognition.)
Web Speech Concepts and Usage
The Web Speech API makes web apps able to handle voice data. There are two components to this API:
- Speech recognition is accessed via the SpeechRecognition interface, which provides the ability to recognize voice context from an audio input (normally via the device's default speech recognition service) and respond appropriately. Generally you'll use the interface's constructor to create a new SpeechRecognition object, which has a number of event handlers available for detecting when speech is input through the device's microphone. The SpeechGrammar interface represents a container for a particular set of grammar that your app should recognize. Grammar is defined using JSpeech Grammar Format ( JSGF .)
- Speech synthesis is accessed via the SpeechSynthesis interface, a text-to-speech component that allows programs to read out their text content (normally via the device's default speech synthesizer.) Different voice types are represented by SpeechSynthesisVoice objects, and different parts of text that you want to be spoken are represented by SpeechSynthesisUtterance objects. You can get these spoken by passing them to the SpeechSynthesis.speak() method.
For more details on using these features, see Using the Web Speech API .
Web Speech API Interfaces
Speech recognition.
The controller interface for the recognition service; this also handles the SpeechRecognitionEvent sent from the recognition service.
Represents a single word that has been recognized by the speech recognition service.
Represents error messages from the recognition service.
The event object for the result and nomatch events, and contains all the data associated with an interim or final speech recognition result.
The words or patterns of words that we want the recognition service to recognize.
Represents a list of SpeechGrammar objects.
Represents a single recognition match, which may contain multiple SpeechRecognitionAlternative objects.
Represents a list of SpeechRecognitionResult objects, or a single one if results are being captured in continuous mode.
Speech synthesis
The controller interface for the speech service; this can be used to retrieve information about the synthesis voices available on the device, start and pause speech, and other commands besides.
Contains information about any errors that occur while processing SpeechSynthesisUtterance objects in the speech service.
Contains information about the current state of SpeechSynthesisUtterance objects that have been processed in the speech service.
Represents a speech request. It contains the content the speech service should read and information about how to read it (e.g. language, pitch and volume.)
Represents a voice that the system supports. Every SpeechSynthesisVoice has its own relative speech service including information about language, name and URI.
Specified out as part of a [NoInterfaceObject] interface called SpeechSynthesisGetter , and Implemented by the Window object, the speechSynthesis property provides access to the SpeechSynthesis controller, and therefore the entry point to speech synthesis functionality.
For information on errors reported by the Speech API (for example, "language-not-supported" and "language-unavailable" ), see the following documentation:
- error property of the SpeechRecognitionErrorEvent object
- error property of the SpeechSynthesisErrorEvent object
The Web Speech API examples on GitHub contains demos to illustrate speech recognition and synthesis.
Specifications
Specification |
---|
Browser compatibility
Api.speechrecognition.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.

api.SpeechSynthesis
- Using the Web Speech API
- SitePoint article
- HTML5Rocks article
- Español – América Latina
- Português – Brasil
- Tiếng Việt
Using the Speech-to-Text API with Node.js
1. overview.
Google Cloud Speech-to-Text API enables developers to convert audio to text in 120 languages and variants, by applying powerful neural network models in an easy to use API.
In this codelab, you will focus on using the Speech-to-Text API with Node.js. You will learn how to send an audio file in English and other languages to the Cloud Speech-to-Text API for transcription.
What you'll learn
- How to enable the Speech-to-Text API
- How to Authenticate API requests
- How to install the Google Cloud client library for Node.js
- How to transcribe audio files in English
- How to transcribe audio files with word timestamps
- How to transcribe audio files in different languages
What you'll need
- A Google Cloud Platform Project
- A Browser, such Chrome or Firefox
- Familiarity using Javascript/Node.js
How will you use this tutorial?
How would you rate your experience with node.js, how would you rate your experience with using google cloud platform services, 2. setup and requirements, self-paced environment setup.
- Sign in to Cloud Console and create a new project or reuse an existing one. (If you don't already have a Gmail or G Suite account, you must create one .)
Remember the project ID, a unique name across all Google Cloud projects (the name above has already been taken and will not work for you, sorry!). It will be referred to later in this codelab as PROJECT_ID .
- Next, you'll need to enable billing in Cloud Console in order to use Google Cloud resources.
Running through this codelab shouldn't cost much, if anything at all. Be sure to to follow any instructions in the "Cleaning up" section which advises you how to shut down resources so you don't incur billing beyond this tutorial. New users of Google Cloud are eligible for the $300USD Free Trial program.
Start Cloud Shell
While Google Cloud can be operated remotely from your laptop, in this codelab you will be using Google Cloud Shell , a command line environment running in the Cloud.
Activate Cloud Shell
If you've never started Cloud Shell before, you'll be presented with an intermediate screen (below the fold) describing what it is. If that's the case, click Continue (and you won't ever see it again). Here's what that one-time screen looks like:
It should only take a few moments to provision and connect to Cloud Shell.
This virtual machine is loaded with all the development tools you'll need. It offers a persistent 5GB home directory and runs in Google Cloud, greatly enhancing network performance and authentication. Much, if not all, of your work in this codelab can be done with simply a browser or your Chromebook.
Once connected to Cloud Shell, you should see that you are already authenticated and that the project is already set to your project ID.
- Run the following command in Cloud Shell to confirm that you are authenticated:
Command output
If it is not, you can set it with this command:
3. Enable the Speech-to-Text API
Before you can begin using the Speech-to-Text API, you must enable the API. You can enable the API by using the following command in the Cloud Shell:
4. Authenticate API requests
In order to make requests to the Speech-to-Text API, you need to use a Service Account . A Service Account belongs to your project and it is used by the Google Client Node.js library to make Speech-to-Text API requests. Like any other user account, a service account is represented by an email address. In this section, you will use the Cloud SDK to create a service account and then create credentials you will need to authenticate as the service account.
First, set an environment variable with your PROJECT_ID which you will use throughout this codelab, if you are using Cloud Shell this will be set for you:
Next, create a new service account to access the Speech-to-Text API by using:
Next, create credentials that your Node.js code will use to login as your new service account. Create these credentials and save it as a JSON file ~/key.json by using the following command:
Finally, set the GOOGLE_APPLICATION_CREDENTIALS environment variable, which is used by the Speech-to-Text API Node.js library, covered in the next step, to find your credentials. The environment variable should be set to the full path of the credentials JSON file you created, by using:
You can read more about authenticating the Speech-to-Text API .
5. Install the Google Cloud Speech-to-Text API client library for Node.js
First, create a project that you will use to run this Speech-to-Text API lab, initialize a new Node.js package in a folder of your choice:
NPM asks several questions about the project configuration, such as name and version. For each question, press ENTER to accept the default values. The default entry point is a file named index.js .
Next, install the Google Cloud Speech library to the project:
For more instructions on how to set up a Node.js development for Google Cloud please see the Setup Guide .
Now, you're ready to use Speech-to-Text API!
6. Transcribe Audio Files
In this section, you will transcribe a pre-recorded audio file in English. The audio file is available on Google Cloud Storage.
Navigate to the index.js file inside the and replace the code with the following:
Take a minute or two to study the code and see it is used to transcribe an audio file*.*
The Encoding parameter tells the API which type of audio encoding you're using for the audio file. Flac is the encoding type for .raw files (see the doc for encoding type for more details).
In the RecognitionAudio object, you can pass the API either the uri of our audio file in Cloud Storage or the local file path for the audio file. Here, we're using a Cloud Storage uri.
Run the program:
You should see the following output:
7. Transcribe with word timestamps
Speech-to-Text can detect time offset (timestamp) for the transcribed audio. Time offsets show the beginning and end of each spoken word in the supplied audio. A time offset value represents the amount of time that has elapsed from the beginning of the audio, in increments of 100ms.
Take a minute or two to study the code and see it is used to transcribe an audio file with word timestamps*.* The EnableWordTimeOffsets parameter tells the API to enable time offsets (see the doc for more details).
Run your program again:
8. Transcribe different languages
Speech-to-Text API supports transcription in over 100 languages! You can find a list of supported languages here .
In this section, you will transcribe a pre-recorded audio file in French. The audio file is available on Google Cloud Storage.
Run your program again and you should see the following output:
This is a sentence from a popular French children's tale .
For the full list of supported languages and language codes, see the documentation here .
9. Congratulations!
You learned how to use the Speech-to-Text API using Node.js to perform different kinds of transcription on audio files!
To avoid incurring charges to your Google Cloud Platform account for the resources used in this quickstart:
- Go to the Cloud Platform Console .
- Select the project you want to shut down, then click ‘Delete' at the top: this schedules the project for deletion.
- Google Cloud Speech-to-Text API: https://cloud.google.com/speech-to-text/docs
- Node.js on Google Cloud Platform: https://cloud.google.com/nodejs/
- Google Cloud Node.js client: https://googlecloudplatform.github.io/google-cloud-node/
This work is licensed under a Creative Commons Attribution 2.0 Generic License.
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Javascript Text to speech library
Hagsten/Talkify
Folders and files.
Name | Name | |||
---|---|---|---|---|
336 Commits | ||||
Repository files navigation
A javascript text to speech (TTS) library. Originally from and used by https://talkify.net .
Give a voice to your website in a matter of minutes. Talkify library provides you with high quality text to speech (TTS) voices in many languages.
To use our backend services (our hosted voices) you will require an api-key. Visit our portal ( https://manage.talkify.net ) to create your own API-key, Talkify offers 1000 free requests per month.
Dependencies
Configuration.
- Form reader
Text selection reader
Installation.
Font Awesome 5+ (Used in Talkify Control Center)
Quick demos
- Web Reader http://jsfiddle.net/5atrbjc6/
- Form Reader http://jsfiddle.net/dx53bg6k/2/
- Text selection Reader http://jsfiddle.net/t5dbcL64/
- Enhanced text visibility http://jsfiddle.net/pwbqkzxj/2/
Include the scripts and stylesheets
Minified version, non-minified version, stylesheets.
You find our stylesheets under /styles folder. Include the stylesheets that you need (i.e. all under /modern-control-center for our "modern" UI).
Play all, top to bottom
Play simple text.
High qualiy voices ( https://manage.talkify.net/docs#voices )
Supported languages:
Text highligting for easy read along
Control pitch, pauses between words, volume, speech rate, phonation and much more
Download as mp3
Playback of entire website or paragraph/s of your choice
Fully integrated UI options
Read web forms aloud
Listen to selected text
Enhanced visibility features
When useSSML is active, Talkify will translate the following markup into SSML. This has the potential of creating a smoother voice experience.
HTML tags | SSML |
---|---|
h1 - h3 | emphasis strong |
b | emphasis strong |
strong | emphasis strong |
i | emphasis reduced |
em | emphasis strong |
br | break-strength strong |
Declarative settings
These settings are only supported by the TtsPlayer for now.
Talkify supports declarative settings. These settings will override general settings. The following attributes can be added to any element that Talkify is connected to. When these attributes are present, Talkify will use them as playback settings.
data-attribute | Accepted values | Example | Remarks |
---|---|---|---|
data-talkify-wordbreakms | [0, 10000] | data-talkify-wordbreakms="100" | |
data-talkify-pitch | [-5, 5] | data-talkify-pitch="-2" | |
data-talkify-rate | [-10, 10] | data-talkify-rate="-2" | |
data-talkify-voice | Any authorized voice | data-talkify-voice="David" | |
data-talkify-phonation | "soft", "normal" or "" | data-talkify-phonation="soft" | |
data-talkify-whisper | "true" or "false" | data-talkify-whisper="true" | |
data-talkify-whisper | "true" or "false" | data-talkify-whisper="true" | |
data-talkify-read-as-lowercase | "true" | data-talkify-read-as-lowercase="true" | Some voices spell out capital letters, which might be unwanted, this setting will read the content of the element as lower case |
WebReader demo
Talkify lives in its own namespace - talkify. Hence, everything below is scoped to that namespace (i.e. talkify.playlist, etc).
Auto scroll
Talkify provides an opt in auto scroll to the item to be played.
Activate the feature by calling talkify.autoScroll.activate()
| Method | | activate |
Playlist fluent builder
Playlist builder is Talkifys way to instantiate your playlist. It comes with a fluent API.
Entry point: talkify.playlist()
Method | Parameters | Default | Description | Mandatory |
---|---|---|---|---|
begin | Entry point. Call this to start building your playlist | Yes | ||
usingPlayer | TtsPlayer/Html5Player | Specify which player to be used. | Yes | |
withTextInteraction | Enables you to click on paragraphs (and other text) to play | No | ||
withElements | DOM elements | Specifies with elements to play. If omitted, Talkify will crawl the page and select for you | No | |
excludeElements | Array of DOM-elements | [] | For example: document.querySelectorAll("button") | No |
withTables | Table configuration, array of objects* | Reads tables in a more intuitive way. The relevant header is repeated before each cell | No | |
withRootSelector | string | 'body' | Sets the scope from where Talkify will start to crawl the page for text to play | No |
subscribeTo | Json object | Event subscriptions | No | |
build | Finalizes and creates the playlist instance | Yes |
*withTables parameter is an array of objects with the following properties:
- table (DOM-query selector or actual DOM-elements)
- headerCells (Optional. DOM-query selector or actual DOM-elements. Defaults to "th")
- bodyCells (Optional. DOM-query selector or actual DOM-elements. Defaults to "td")
withTables works with any standard HTML-table and other non-standard tabular content (for example bootstrap grid system). For non standard tabular content, please use the optional parameters to tell Talkify which elements are header cells and which are body cells.
This is the instance built from the playliste above.
Method | Parameters | Default | Description |
---|---|---|---|
getQueue | Returns the playlist queue | ||
play | Begins playback of playlist | ||
pause | Pauses playlist | ||
replayCurrent | Replays the current item in the playlist | ||
insert | DOM element | Inserts new html elements to play. Useful for elements that Talkify were unable to locate. Elements will be inserted in correct order with respect to the page. | |
isPlaying | True if any item is currently in a playing state | ||
setPlayer | TtsPlayer/Html5Player | Sets the player that the playlist is using | |
enableTextInteraction | Enables click to play on HTML elements | ||
disableTextInteraction | Disables click to play on HTML elements | ||
dispose | Clean up |
Playlist Events
Event |
---|
onEnded |
onVoiceCommandListeningStarted |
onVoiceCommandListeningEnded |
Player (valid for all players)
Method | Parameters | Default | Description |
---|---|---|---|
enableTextHighlighting | Tells the player to use text highlighting. For Html5Player this only works on localVoice. | ||
disableTextHighlighting | Turns off text highlighting. | ||
subscribeTo | Json object | Event listeners | |
playText | string | Plays a text | |
paused | True if paused | ||
isPlaying | True if playing | ||
play | Play | ||
pause | Pause | ||
forceVoice | object | For Talkify hosted voices, this is a JSON object with a name property. The value of name should be the name of a voice from /api/speech/v1/voices. For browser voices, this is the actual voice from window.speechSynthesis.getVoices() | |
enableEnhancedTextVisibility | Enables enhanced text visibility. Subtitle-bar, with a larger font-size, is added to the bottom of the screen. | ||
disableEnhancedTextVisibility | Disables enhanced text visibility |
Html5Player only
Entry point: talkify.Html5Player().
Method | Parameters | Default | Description |
---|---|---|---|
forceLanguage | string | Force the usage of a specific language. Use standard cultures like se-SE for Swedish and so on. Talkify will select a voice that matches the culture. | |
setRate | double | 1 | [0.0, 2.0] Playback rate. |
setVolume | double | 1 | [0.0 - 1.0 ] |
usePitch | double | 1 | [0.0, 2.0] Adjusts the pitch of the voice. |
Talkify hosted only
Entry point: talkify.TtsPlayer(options?).
constructor parameter "options" is optional. Example { controlcenter: { container: document.querySelector('p.selector') , name: 'modern' }}
Method | Parameters | Default | Description |
---|---|---|---|
setRate | int | 1 | Playback rate. A value between -5 and 5 |
whisper | Sets the player to whispering mode | ||
normalTone | Sets the player to normal mode (opposite of whispering) | ||
usePhonation | string | normal | Supports for two phonations. "soft" and "normal". Empty string translates to "normal". Case sensitive |
useWordBreak | int | 0 | [0-10000] Adds a break between each word. Any value above 0 adds to the voices standard break length. |
usePitch | int | 0 | [-10 - +10] Adjusts the pitch of the voice. |
useVolumeBaseline | double | 0 | [-10 - +10] Adjusts the volume baseline |
Player Events
Event |
---|
onBeforeItemPlaying |
onSentenceComplete |
onPause |
onPlay |
onResume |
onItemLoaded |
onTextHighligtChanged |
Example: talkify.formReader.addForm(document.getElementById("form-id"));
Method | Parameters | Default | Description |
---|---|---|---|
addForm | form element | None | Adds TTS functionality to the form. |
removeForm | form element | None | Unbinds all TTS functionality from the form |
This feature allows the user to select/mark text using the mouse and have that text read aloud.
Method | Parameters | Default | Description |
---|---|---|---|
activate | - | - | Call this method to actiate the feature |
deactivate | - | - | Call this method to deactivate the feature |
withTextHighlighting | - | - | Presets text highlighting to activated. Users can turn this off in the control center UI |
withEnhancedVisibility | - | - | Presets enhanced visibility to activated. Users can turn this off in the control center UI |
withVoice | voice object | { name: 'Zira' } | A voice object from our backend voice API or at the very least an object wih a name property including a valid voice name |
withButtonText | string | "Listen" | The text that appears on popover button |
excludeElements | Array of DOM-elements | [] | For example: document.querySelectorAll("button") |
React to events
TLDR; Example @ http://jsfiddle.net/andreas_hagsten/x6pve0jd/8/
Talkify provides two event models - PubSub and classic callbacks. The newest, and primary, model is the PubSub model. PubSub is a loosly coupled model which enables client applications to hook in to the Talkify pipeline. To subscribe to events you will need to pass a context key (used when unsubscribing) as well as the event type and the event handler function. The event type is a string containing topics. An event is normally divided into 4 topics - context, origin, type and action.
The Context topic
You would use this top level topic if you run multiple instances of Talkify. This allows you to hook into a specific Talkify instance. If you want to listen to all instances or only have one just specify "*". You will find the context ID in the property "correlationId" of your Player instance.
The Origin topic
Where the event originates from. For example "player" or "controlcenter". A common use case is to listen to player events which is done by specifying "player" in this topic section.
The type topic
Type of event. For example "tts" for TTS-based events.
The action topic
This is the topic that describes what action is taken. This can be "play", "loading", "pause" and so forth.
Putting all 4 topics together forms the event type to listen to. You can replace any part with the wildcard "*" which means that you listens to all events of the given topic.
A few examples can be seen below. A full list of events supported is listed Here .
PubSub events
Type | args (TBD) |
---|---|
{contextId}.player.tts.ratechanged | |
{contextId}.player.tts.seeked | |
{contextId}.player.tts.pause | |
{contextId}.player.tts.timeupdated | |
{contextId}.player.tts.play | |
{contextId}.player.tts.resume | |
{contextId}.player.tts.loading | |
{contextId}.player.tts.loaded | |
{contextId}.player.tts.ended | |
{contextId}.player.tts.voiceset | |
{contextId}.player.tts.texthighlight.enabled | |
{contextId}.player.tts.texthighlight.disabled | |
{contextId}.player.tts.prepareplay | |
{contextId}.player.tts.disposed | |
{contextId}.player.tts.error | |
{contextId}.player.tts.phonationchanged | |
{contextId}.player.tts.whisperchanged | |
{contextId}.player.tts.wordbreakchanged | |
{contextId}.player.tts.volumechanged | |
{contextId}.player.tts.pitchchanged | |
{contextId}.player.tts.created | |
{contextId}.player.tts.unplayable | |
{contextId}.player.tts.enhancedvisibilityset | |
{contextId}.player.tts.creating | |
- | - |
{contextId}.player.html5.ratechanged | |
{contextId}.player.html5.pause | |
{contextId}.player.html5.utterancecomplete | |
{contextId}.player.html5.ended | |
{contextId}.player.html5.loaded | |
{contextId}.player.html5.play | |
{contextId}.player.html5.timeupdated | |
{contextId}.player.html5.voiceset | |
{contextId}.player.html5.texthighlight.enabled | |
{contextId}.player.html5.texthighlight.disabled | |
{contextId}.player.html5.prepareplay | |
{contextId}.player.html5.created | |
{contextId}.player.html5.unplayable | |
{contextId}.player.html5.enhancedvisibilityset | |
{contextId}.player.html5.creating | |
- | - |
{contextId}.controlcenter.request.play | |
{contextId}.controlcenter.request.pause | |
{contextId}.controlcenter.request.rate | |
{contextId}.controlcenter.request.volume | |
{contextId}.controlcenter.request.pitch | |
{contextId}.controlcenter.request.wordbreak | |
{contextId}.controlcenter.request.phonation.normal | |
{contextId}.controlcenter.request.phonation.soft | |
{contextId}.controlcenter.request.phonation.whisper | |
{contextId}.controlcenter.request.texthighlightoggled | |
{contextId}.controlcenter.request.textinteractiontoggled | |
{contextId}.controlcenter.request.enhancedvisibility | |
{contextId}.controlcenter.attached | |
{contextId}.controlcenter.detached | |
- | - |
{contextId}.wordhighlighter.complete | |
- | - |
{contextId}.playlist.playing | |
{contextId}.playlist.loaded | |
{contextId}.playlist.textinteraction.enabled | |
{contextId}.playlist.textinteraction.disabled |
Releases 42
Used by 152.
Contributors 2
- JavaScript 96.0%
- About AssemblyAI
JavaScript Text-to-Speech - The Easy Way
Learn how to build a simple JavaScript Text-to-Speech application using JavaScript's Web Speech API in this step-by-step beginner's guide.

Contributor
When building an app, you may want to implement a Text-to-Speech feature for accessibility, convenience, or some other reason. In this tutorial, we will learn how to build a very simple JavaScript Text-to-Speech application using JavaScript's built-in Web Speech API .
For your convenience, we have provided the code for this tutorial application ready for you to fork and play around with over at Replit , or ready for you to clone from Github . You can also view a live version of the app here .
Step 1 - Setting Up The App
First, we set up a very basic application using a simple HTML file called index.html and a JavaScript file called script.js .
We'll also use a CSS file called style.css to add some margins and to center things, but it’s entirely up to you if you want to include this styling file.
The HTML file index.html defines our application's structure which we will add functionality to with the JavaScript file. We add an <h1> element which acts as a title for the application, an <input> field in which we will enter the text we want spoken, and a <button> which we will use to submit this input text. We finally wrap all of these objects inside of a <form> . Remember, the input and the button have no functionality yet - we'll add that in later using JavaScript.
Inside of the <head> element, which contains metadata for our HTML file, we import style.css . This tells our application to style itself according to the contents of style.css . At the bottom of the <body> element, we import our script.js file. This tells our application the name of the JavaScript file that stores the functionality for the application.
Now that we have finished the index.html file, we can move on to creating the script.js JavaScript file.
Since we imported the script.js file to our index.html file above, we can test its functionality by simply sending an alert .
To add an alert to our code, we add the line of code below to our script.js file. Make sure to save the file and refresh your browser, you should now see a little window popping up with the text "It works!".
If everything went ok, you should be left with something like this:

Add Speech Recognition to Your JavaScript App
Get started with AssemblyAI's powerful JavaScript SDK for free.
Step 2 - Checking Browser Compatibility
To create our JavaScript Text-to-Speech application, we are going to utilize JavaScript's built-in Web Speech API. Since this API isn’t compatible with all browsers, we'll need to check for compatibility. We can perform this check in one of two ways.
The first way is by checking our operating system and version on caniuse.com .
The second way is by performing the check right inside of our code, which we can do with a simple conditional statement:
This is a shorthand if/else statement, and is equivalent to the following:
If you now run the app and check your browser console, you should see one of those messages. You can also choose to pass this information on to the user by rendering an HTML element.
Step 3 - Testing JavaScript Text-to-Speech
Next up, let’s write some static code to test if we can make the browser speak to us.
Add the following code to the script.js file.
Code Breakdown
Let’s look at a code breakdown to understand what's going on:
- With const synth = window.speechSynthesis we declare the synth variable to be an instance of the SpeechSynthesis object, which is the entry to point to using JavaScript's Web Speech API. The speak method of this object is what ultimately converts text into speech.
- let ourText = “Hey there what’s up!!!!” defines the ourText variable which holds the string of text that we want to be uttered.
- const utterThis = new SpeechSynthesisUtterance(ourText) defines the utterThis variable to be a SpeechSynthesisUtterance object, into which we pass ourText .
- Putting it all together, we call synth.speak(utterThis) , which utters the string inside ourText .
Save the code and refresh the browser window in which your app runs in order to hear a voice saying “ Hey there what’s up!!!! ”.
Experience Speech-to-Text in Action
Test our API with your own audio files in our interactive playground - no coding required.
Step 4 - Making Our App Dynamic
Our code currently provides us with a good understanding of how the Text-to-Speech aspect of our application works under the hood, but the app at this point only converts the static text which we defined with ourText into speech. We want to be able to dynamically change what text is being converted to speech when using the application. Let’s do that now utilizing a <form> .
- First, we add the const textInputField = document.querySelector("#text-input") variable, which allows us to access the value of the <input> tag that we have defined in the index.html file in our JavaScript code. We select the <input> field by its id: #text-input .
- Secondly, we add the const form = document.querySelector("#form") variable, which selects our form by its id #form so we can later submit the <form> using the onsubmit function.
- We initialize ourText as an empty string instead of a static sentence.
- We wrap our browser compatibility logic in a function called checkBrowserCompatibility and then immediately call this function.
Finally, we create an onsubmit handler that executes when we submit our form. This handler does several things:
- event.preventDefault() prevents the browser from reloading after submitting the form.
- ourText = textInputField.value sets our ourText string to whatever we enter in the "input" field of our application.
- utterThis.text = ourText sets the text to be uttered to the value of ourText .
- synth.speak(utterThis) utters our text string.
- textInputField.value resets the value of our input field to an empty string after submitting the form.
Step 5 - Testing Our JavaScript Text-to-Speech App
To test our JavaScript Text-to-Speech application, simply enter some text in the input field and hit “Submit” in order to hear the text converted to speech.
Additional Features
There are a lot of properties that can be modified when working with the Web Speech API. For instance:
You can try playing around with these properties to tailor the application to your needs.
This simple example provides an outline of how to use the Web Speech API for JavaScript Text-to-Speech .
While Text-to-Speech is useful for accessibility, convenience, and other purposes, there are a lot of use-cases in which the opposite functionality, i.e. Speech-to-Text, is useful. We have built a couple of example projects using AssemblyAI’s Speech-to-Text API that you can check out for those who want to learn more.
Ready to Add Speech-to-Text to Your JavaScript Project?
Sign up for a free AssemblyAI account and get started with our powerful Speech-to-Text API.
Some of them are:
- React Speech Recognition with React Hooks
- How To Convert Voice To Text Using JavaScript
Popular posts

How to identify languages in audio data using Python

Senior Developer Advocate

Speech AI apps: 8 new Speech AI tools, releases, updates, and more
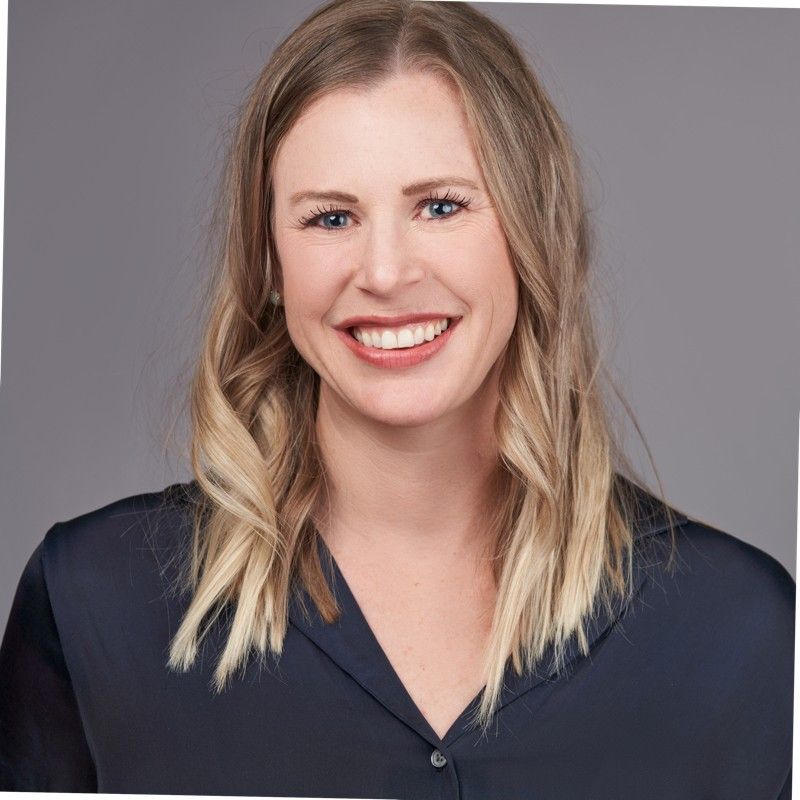
How to perform Speaker Diarization in Python
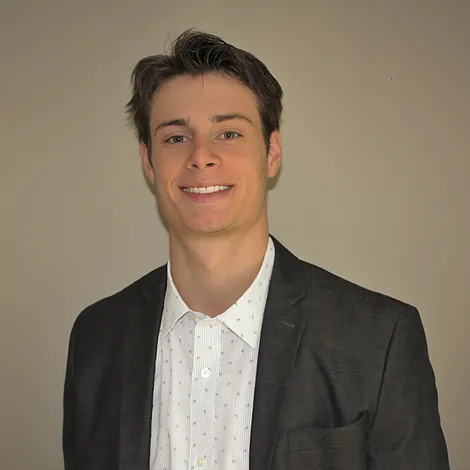
Developer Educator

Speaker diarization vs speaker recognition - what's the difference?

16 Open-source Web-based Text-to-Speech Apps and TTS JavaScript Libraries
Hazem Abbas
Open-source JavaScript libraries are ideal sources to build rich applications.
In recent years, we have seen a substantial increase in the demand for text-to-speech (TTS) technology. This technology is an excellent example of assistive technology that has been developed to help individuals with visual impairments and those who have difficulty reading by converting text into spoken language. The use of TTS technology has been instrumental in providing equal opportunities for people of different abilities to access the same information available to others.
JavaScript libraries have emerged as a popular and accessible way to implement TTS on web-based applications, making it easier for developers to create more inclusive websites and applications that cater to a wider audience. By using these libraries, web developers can incorporate TTS functionality into their applications without the need for complex and time-consuming coding, saving them valuable time and resources. Moreover, the availability of these libraries has made TTS more accessible to developers who are not proficient in programming, thus making it easier for them to create applications that are accessible to everyone.
Cloud-based TTS Services
There are several open-source TTS JavaScript libraries available today, each with its own unique features and advantages. Here are some of the most popular options:
- ResponsiveVoice.js: This library is easy to integrate and has a wide range of voice options and languages. It also includes a customizable player for users to control the speech.
- Speak.js: This library is lightweight and fast, making it ideal for smaller projects. It also supports multiple languages and voices.
- Pico TTS: This library is very small and can be used on low-powered devices. It has a limited set of voices but is suitable for basic TTS projects.
- Amazon Polly: This library is not strictly JavaScript, but it has a JavaScript SDK for easy integration. It offers a wide range of high-quality voices and languages, but it requires an AWS account and has associated costs.
- Google Cloud Text-to-Speech: Similar to Amazon Polly, this library has a JavaScript SDK and offers high-quality voices and languages. It also requires a Google Cloud account and has associated costs.
Open-source JavaScript Libraries
Open-source TTS JavaScript libraries have made it easier than ever for developers to implement TTS technology into their web-based applications. With the variety of options available, developers can choose the library that best suits their needs and create more accessible applications for users.
Lizen is a text to speech web application that speaks word, sentences or even reads long articles in a music player like interface. It can also fetch articles from a link or text can be directly pasted.
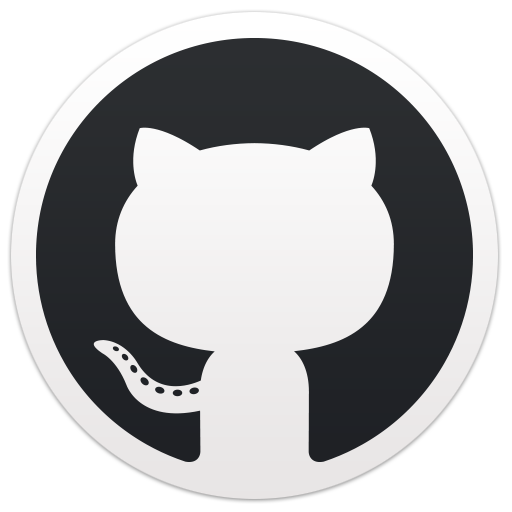
2- ttspeech
ttspeech is a free open-source basic voice synthesizer apps written in vanillaJS.
3- <k-speech>
This one is a free open-source simple web component " text to speech ".
4- Text-Editor-React
This project is a complete multi-tool web app written using React. It includes Text Editor, Text-to-speech, Analysis, Summary and many more.
5- ONLINE TTS
Online TTS is a free text to speech converter. Powered by ResponsiveVoice.JS.
6- Read aloud
Read Aloud is a Chrome and Firefox extension that uses text-to-speech technology to convert webpage text to audio. It works on a variety of websites, including news sites, blogs, fan fiction, publications, textbooks, school and class websites, online universities and course materials.
7- Voice Builder
Voice Builder is an open source text-to-speech (TTS) voice building tool that focuses on simplicity, flexibility, and collaboration. Our tool allows anyone with basic computer skills to run voice training experiments and listen to the resulting synthesized voice.
8- Google TTS
Google TTS (Text-To-Speech) library for node.js
9- TTS Web app
This web app will convert your text to speech using voices provided by a variety of TTS services including Amazon Polly, CereProc, IBM Watson, Acapela, Oddcast, ReadSpeaker, Google Translate, iSpeech.
This one is a Google Chrome extension that converts any selected text on the web to speech.
11- Click to Read - TTS (Chrome)
Another Google Chrome extension designed for minimal clicks or presses to start reading text out loud.
12- Speakable
Speakable app is a simple and privacy-friendly on-page screen reader / text-to-speech (TTS) player using the native JavaScript Web Speech API.
13- Voice Builder by Google
Voice Builder is an open-source text-to-speech (TTS) voice building tool that focuses on simplicity, flexibility, and collaboration. Our tool allows anyone with basic computer skills to run voice training experiments and listen to the resulting synthesized voice.
14- tts-react
tts-react is a React library that provides a hook ( useTts ) and component ( TextToSpeech ) to convert text to speech. In most cases you want the hook so you can use custom styling on the audio controls.
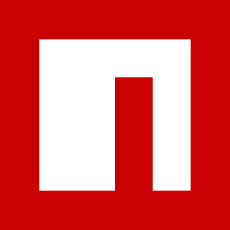
15- React speech recognition and speech synthesis
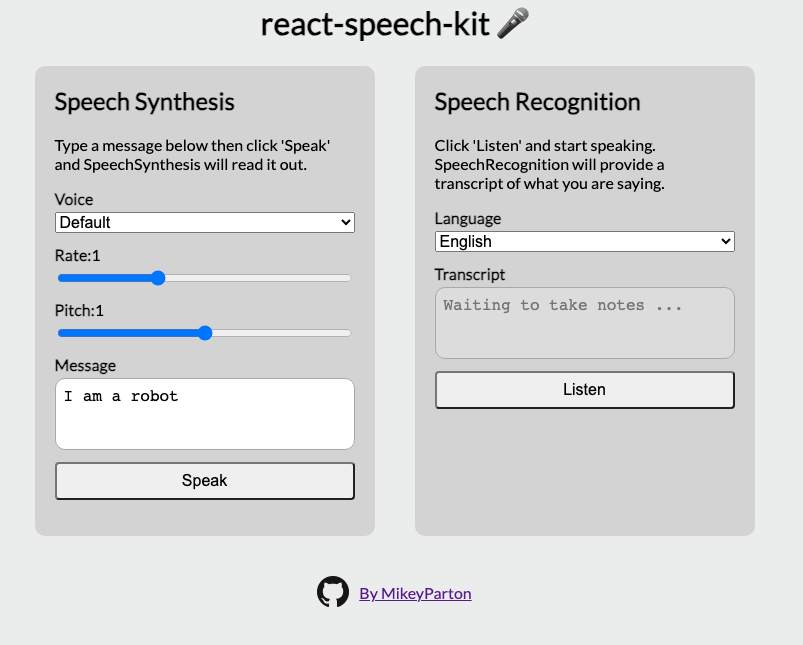
React hooks for in-browser Speech Recognition and Speech Synthesis.
16- React Speech Highlight
This open-source project offers a React components that use web speech synthesis API to text-to-speech tasks and also highlight the word and sentences that are being spoken. You can check the demo here .
Read More Articles in tts
Echocharm is a free versatile text-to-speech tts application, "audapolis": the revolutionary editor empowering spoken-word media editing, koodo reader: open-source ebook reader (free app), 16 open-source and free tts (text-to-speech) programs for windows, best 10 free text to speech (tts) services, top 15 resources for free tailwind components: access 5500+ open-source ui elements to enhance your frontend development.
What Are Tailwind Components? Tailwind components are pre-designed, reusable UI elements built with Tailwind CSS—a utility-first CSS framework that enables developers to create custom designs directly in their HTML. These components, such as buttons, navigation bars, modals, and forms, serve as ready-made building blocks. Developers can quickly integrate them
300+ Free Tailwind Components: A Game Changer for Front-End and React Developers
Tailwind Components Repo is a remarkable collection of pre-designed, customizable elements built with Tailwind CSS. It caters specifically to front-end and React developers, streamlining the process of designing responsive user interfaces while maintaining flexibility and scalability. This makes it an essential tool for those working on React and Next.js
Flowrift: The Premier Free Source for Responsive Tailwind CSS Snippets
In web development, finding the right components and snippets can significantly streamline your workflow. Flowrift, a Tailwind CSS snippets source, offers developers a solution to accelerate their design and build processes. Whether you're creating a website from scratch or enhancing existing projects, Flowrift provides an extensive collection of
AlertR: The Ultimate Multi-Purpose Alarm and Monitoring System for Developers and Tinkerers
AlertR is a client-server-based, multi-purpose alarm, monitoring, and signaling system designed for developers, privacy advocates, and DIY enthusiasts who demand full control over their data and systems. Whether you're setting up a home alarm system, monitoring servers, or managing complex sensor-based automation, AlertR offers the flexibility and functionality
Development
Science - healthcare, open-source apps, medical apps, dev. resources.

✔️ Safe Payments ✔️ 51 Languages ✔️ Free Trial
Lifelike AI Voices
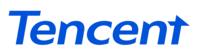
MAKE MY WEBSITE SPEAK IN 3 MINUTES!
Easily add AI voice features to your site in 51 languages in a matter of minutes with the most popular HTML5 text-to-speech API. Includes a voice message editor, accessibility compliance features, a developer API and it’s free for non-commercial sites .
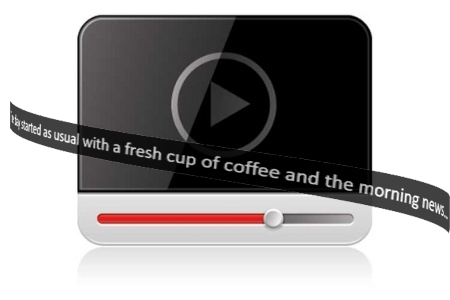
HOW TO CREATE AI VOICE-OVERS FOR MY VIDEOS
Add voices to any video. Generate semi-automatic voice-overs for your videos from text, in 13 languages. Use a YouTube URL or upload your own MP4 and apply text-to-speech in the easy to use editor.
WHAT ARE YOU LOOKING FOR?
ResponsiveVoice is not built for this purpose, however if you host your text or notes as webpages on your own website you can add ResponsiveVoice to your site and listen content that way.
ResponsiveVoice supports reading HTML webpages, you may convert your documents or PDFs to webpages on your own website and then add ResponsiveVoice to your site as a solution.
ResponsiveVoice generates AI speech in real-time, it does not generate mp3 or downloadable audio files. Just add the ResponsiveVoice script to your blog and you can have any blog page or post spoken out loud.
You can create AI voice overs for videos here Text2VoiceOver
ResponsiveVoice is built specifically for this case, sign-up for the free service, get your unique code and add it to your website to instantly enable an AI voice for your website visitors.
ResponsiveVoice is created for website owners to add AI voice features to their own site. It is not a tool to read every website you visit while browsing.
ResponsiveVoice is perfect for use with queue management systems for announcing tickets with an AI voice. ResponsiveVoice is a text-to-speech library. Contact us if you have a specific need for speech recognition or speech-to-text. ResponsiveVoice is perfect for use with queue management systems for announcing tickets with an AI voice.
ResponsiveVoice is a JavaScript library, it will work in any WebView in an App. ResponsiveVoice does require an internet connection to operate.
ResponsiveVoice is the perfect choice for quickly adding accessibility AI voice features to your website. Sign-up, add the unique code to your website and allow all your visitors to enjoy your content.
ResponsiveVoice is the prefect solution for having articles read out loud on your own website. Text to speech is generated in real-time so no transmission or management of audio files is needed. It couldn’t be simpler!
ResponsiveVoice is perfect for use with queue management systems for announcing tickets with an AI voice.
Developer API
Add one line of code to your site or app
AI Text-to-Speech for Articulate Storyline & Adobe Captivate
Free for Non-Commercial
Do you want to use ResponsiveVoice for a non-commercial personal or non-profit project?
1. WordPress Plugin (free for commercial and non-commercial use) 2. ResponsiveVoice Free ( feature limited for commercial and free for non-commercial use) 3. AI Voice API (free for non-commercial use only)
All other ResponsiveVoice products require paid licenses.
Just add the attribution to your site:
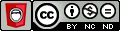
If you have questions about the Non-commercial license contact us
How does it work?
Browser & device support.
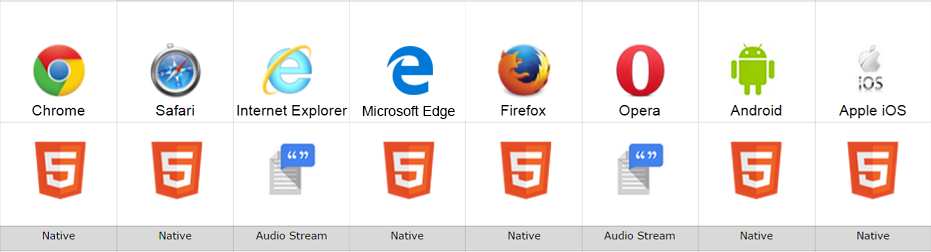
Don’t Clog the Tubes!
HTML5 introduces the Speech API for AI Speech Synthesis and Speech Recognition.
This is the easiest way to use the spoken word in your app or website.
AI Speech Synthesis or more commonly known as Text To Speech (TTS) is now available in most modern browsers.
Gone are the days of waiting for Text To Speech engines to render MP3 audio files from text and then download them from servers.
Today the browser can instantly speak text on the client side and with quite reasonable quality.
Gargling Bagpipes
But there is a problem, each browser and device can have a different set of “AI Voices”. You can’t be sure of a consistent user experience when it comes to the spoken voice or accent.
If you make a call to the AI speak API using the default AI voice it will sound very different on different users devices and browsers. In some cases you won’t even know if the user will get a male or female AI voice.
Although, you make a direct call to the AI speak API and choose a specific voice like “Google UK Female”, if a user is browsing on iOS with Safari the AI voice will not be available.
Responsive Design for AI Voice
Taking inspiration from Responsive Web Design we have created responsivevoice.js a library that can easily be included in a web page that allows you to make simple api calls to speak text.
Responsive AI Voices
ResponsiveVoice JS defines a selection of smart AI Voice profiles that know which AI voice to use for the users device in order to create a consistent experience no matter which browser or device the speech is being spoken on.
By choosing one ResponsiveVoice the closest AI voice is chosen on
- iOS (Safari & Chrome)
- Android (Chrome, Including across the popular Text To Speech engines Ivona, Acapela, Samsung)
- Windows (Chrome Desktop)
- Mac OSX (Safari & Chrome)
Smart Chunking
With large blocks of text ResponsiveVoice splits up the text into chunks, with preference given to splitting at the end of sentences. Preference is given to splitting at full stop, question mark, colon or semi-colon after that split is performed by the nearest comma and falling back from that the nearest space between words.
ResponsiveVoice JS also takes care of a number of hindrances from the various implementations of HTML5 Speech API across browsers and operating systems.
- Chrome desktop has a limit on the number of characters it can speak, under the hood ResponsiveVoice JS automatically chunks text into acceptable blocks
- Chrome desktop will not speak unless initialised after page load, ResponsiveVoice JS resolves this
- iOS Safari & Chrome require timing delays between AI speech API calls, ResponsiveVoice JS resolves this
- iOS TTS can’t be triggered without a direct user interaction, ResponsiveVoice JS resolves this
- Internet Explorer speech rate is slower, ResponsiveVoice JS resolves this
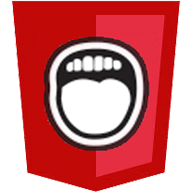
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
A good Text-to-Speech JavaScript library [closed]
I am looking for a good text to speech javascript library for my application. I looked around and saw Jtalk and speak.js . But I'm not sure which one to go forth with. I'm curious to know from people about the pros and cons of them and I'm interested to know if there's any other Javascript plugin you guys came across.
I am basically visualizing a animation and I wanted to add some audio for sight-impaired people to tell them what is happening.
- jquery-plugins
- text-to-speech
- html5-audio
- 4 " for sight impaired people to tell them what is happening" really a good thinking. – Ram Commented Jul 1, 2012 at 3:26
- An interactive demo of speak.js can be found here: jsfiddle.net/YgWdh/30 – Anderson Green Commented Feb 26, 2013 at 6:09
- 1 @AndersonGreen, this demo does not work for me... on Chrome, Windows 8. Microphone works and speakers too, just checked. – Adam Commented Mar 17, 2014 at 21:12
2 Answers 2
UPDATE 2018
Modern browser has built in TTS
speechSynthesis
If you don't want to write a lot of code (or import some library that you don't understand), I suggest you use Google TTS services. It's quite simple. For example you have a text like: welcome to Stack overflow you can call like this:
This will return an audio file reading that text. Then your job is to use browser audio to play it. Nice and easy.
PS: Google TTS has really true voice.
Example: http://jsfiddle.net/qspcL/
Reference from: http://jnjnjn.com/187/playing-audio-on-the-ipad-with-html5-and-javascript/

- The voice looks far better than js plugins. But how responsive would be using this service with my app. will it sync with the speed of my animation, basically I have ~.5 secs between every action. And moreover do you have a sample program for how to make the call to the google tts.Thanks – Ajax3.14 Commented Jul 1, 2012 at 3:40
- 1 thanks a lot!! it works in safari not chrome..Am I missing something – Ajax3.14 Commented Jul 1, 2012 at 4:12
- May be Chrome is missing some audio plugins, I suggest u use SoundManager js library. It will handle alot of things – Nhu Trinh Commented Jul 1, 2012 at 4:14
- 6 This was deprecated at the time and is shut down now. – John McLear Commented Dec 9, 2012 at 22:10
- 1 I worked for me for a few hours and now request to Google end up "pending" and "canceled". I've tried everything but the code worked and now stopped working, so I guess Google only allows few requests to its webservice... – Matthieu Napoli Commented Feb 3, 2013 at 10:17
Well I suggest you use JTtalk. It depends what your target crowd is, because legally blind and what you may think is blind can differ and not everyone knows Braille. The benefits I saw of Jtalk really relied on the examples it had, and how well they worked. To do our entire front-end part of the project with JTalk only took us 2 days, because we pretty much just edited the examples it had. Speak.js had a more difficult learning curve in my/my group's opinion. However, we found that the speech was a bit slurred and had comprehension issues, especially for documents where there were a lot of names (example stock articles mentioning companies). This is why we moved to Braille.
My senior design project was for the blind, however we realized that the text to speech wasn't where we wanted to go because we felt Braille was better. With Braille you can multitask better, easily go back and pause and most blind people can read Braille a lot faster then they can listen.

Not the answer you're looking for? Browse other questions tagged javascript jquery jquery-plugins text-to-speech html5-audio or ask your own question .
- The Overflow Blog
- The evolution of full stack engineers
- One of the best ways to get value for AI coding tools: generating tests
- Featured on Meta
- User activation: Learnings and opportunities
- Site maintenance - Mon, Sept 16 2024, 21:00 UTC to Tue, Sept 17 2024, 2:00...
- Staging Ground Reviewer Motivation
- What does a new user need in a homepage experience on Stack Overflow?
Hot Network Questions
- How much technological progress could a group of modern people make in a century?
- Electrical panel not sending 240
- Is this a misstatement of Euclid in Halmos' Naive Set Theory book?
- Navigating career options after a disastrous PhD performance and a disappointed advisor?
- What is the shortest viable hmac for non-critical applications?
- Was Willy Wonka correct when he accused Charlie of stealing Fizzy Lifting Drinks?
- When deleting attribute from GDB file all the fields in the remaining attributes get deleted as well in QGIS
- Overstaying knowing I have a new Schengen visa
- What about the other 35 children who were born in the same manner in The Umbrella Academy. Do we hear what happened to them in the comic or TV Show?
- Use pgf string as property value
- Is it feasible to create an online platform to effectively teach college-level math (abstract algebra, real analysis, etc.)?
- What are the pros and cons of the classic portfolio by Wealthfront?
- How can a microcontroller (such as an Arduino Uno) that requires 7-21V input voltage be powered via USB-B which can only run 5V?
- Are data in the real world "sampled" in the statistical sense?
- How can I analyze the anatomy of a humanoid species to create sounds for their language?
- Philosophical dogma hindering scientific progress?
- Exam package: \numpages wrong when enforcing an even number of pages
- Working principle of the Zener diode acting as a voltage regulator in a circuit
- Where to put acknowledgments in a math paper
- BASH - Find file with regex - Non-recursively delete number-only filenames in directory
- 4/4 time change to 6/8 time
- When does a finite group have finitely many indecomposable representations?
- Can a V22 Osprey operate with only one propeller?
- Engaging students in the beauty of mathematics

COMMENTS
Using the Web Speech API. The Web Speech API provides two distinct areas of functionality — speech recognition, and speech synthesis (also known as text to speech, or tts) — which open up interesting new possibilities for accessibility, and control mechanisms. This article provides a simple introduction to both areas, along with demos.
Step 1: Set up the HTML code and microphone recorder. Create a file index.html and add some HTML elements to display the text. To use a microphone, we embed RecordRTC, a JavaScript library for audio and video recording. Additionally, we embed index.js, which will be the JavaScript file that handles the frontend part.
A voice control - voice commands - speech recognition and speech synthesis javascript library. Create your own siri,google now or cortana with Google Chrome within your website. ... To associate your repository with the speech-to-text topic, visit your repo's landing page and select "manage topics." Learn more Footer
A text-to-speech converter should have a text area at the top so that, the user can enter a long text to be converted into speech followed by a button that converts the entered text into speech and plays th ... React.js: React is a declarative, efficient, and flexible JavaScript library for building user interfaces. It's 'V' in MVC ...
In this short tutorial, we will build a simple yet useful real-time speech-to-text web app using the Web Speech API. Feature-wise, it will be straightforward: click a button to start recording, and your speech will be converted to text, displayed in real-time on the screen. We'll also play with voice commands; saying "stop recording" will halt ...
Congratulations, you've just created your very own Voice-to-Text web application using JavaScript and the Speech Recognition API! 🎉 Now, when you visit your web page, you can click the ...
With the Web Speech API, we can recognize speech using JavaScript. It is super easy to recognize speech in a browser using JavaScript and then getting the text from the speech to use as user input. We have already covered How to convert Text to Speech in Javascript. But the support for this API is limited to the Chrome browser only. So if you ...
It expects one argument, an instance of the beautifully named SpeechSynthesisUtterance class. Here is the entire code needed to read out a string. function readOutLoud(message) {. var speech = new SpeechSynthesisUtterance(); // Set the text and voice attributes. speech.text = message; speech.volume = 1; speech.rate = 1;
Artyom is a JavaScript SpeechRecognition library that allow you to add voice commands and speech synthesis to your website easily. A speech recognition, voice commands and speech synthesis javascript library. ... Speech text. Use artyom.say to speak text. The language is retrieven at the initialization from the lang property. Note.
If you do not encapsulate speech recognition in a function, the speech will be captured the moment you open the web page. That's not what we want. Next, we are creating a constant variable called SpeechRecognition. This will hold the Speech Recognition interface. It's an interface that will provide the tools we need to capture speech.
A JavaScript text to speech (TTS) library. Provides you with high quality TTS voices in many languages and a high quality language. These voices and engines runs on a Talkify hosted server. This lib also supports browser built in voices via the SpeechSynt. Latest version: 4.0.0, last published: a year ago. Start using talkify-tts in your project by running `npm i talkify-tts`.
Whisper Speech-to-Text is a JavaScript library that allows you to record audio from a user's microphone, and then transcribe the audio into text using OpenAI's Whisper ASR system. This library is designed to be used in web applications.
The words will start printing in HTML as you speak. For ending the speech recognition, simply click the Stop button. As soon as you click it, a new button Save to PDF will appear. This button will convert your speech to PDF and send it to the browser. Create the index.html file and add the following code to it.
Choosing the best Speech-to-Text API, AI model, or open-source engine to build with can be challenging.You need to compare accuracy, model design, features, support options, documentation, security, and more. This post examines the best free Speech-to-Text APIs and AI models on the market today, including ones that have a free tier, to help you make an informed decision.
About. meSpeak.js (modulary enhanced speak.js) is a 100% client-side JavaScript text-to-speech library based on the speak.js project, a port of the eSpeak speech synthesizer from C++ to JavaScript using Emscripten. meSpeak.js adds support for Webkit and Safari and introduces loadable voice modules. Also there is no more need for an embedding ...
The Web Speech API makes web apps able to handle voice data. There are two components to this API: Speech recognition is accessed via the SpeechRecognition interface, which provides the ability to recognize voice context from an audio input (normally via the device's default speech recognition service) and respond appropriately. Generally you'll use the interface's constructor to create a new ...
1. Overview Google Cloud Speech-to-Text API enables developers to convert audio to text in 120 languages and variants, by applying powerful neural network models in an easy to use API.. In this codelab, you will focus on using the Speech-to-Text API with Node.js. You will learn how to send an audio file in English and other languages to the Cloud Speech-to-Text API for transcription.
Talkify. A javascript text to speech (TTS) library. Originally from and used by https://talkify.net. Give a voice to your website in a matter of minutes. Talkify library provides you with high quality text to speech (TTS) voices in many languages. To use our backend services (our hosted voices) you will require an api-key.
Step 2 - Checking Browser Compatibility. To create our JavaScript Text-to-Speech application, we are going to utilize JavaScript's built-in Web Speech API. Since this API isn't compatible with all browsers, we'll need to check for compatibility. We can perform this check in one of two ways.
Open-source JavaScript libraries are ideal sources to build rich applications. In recent years, we have seen a substantial increase in the demand for text-to-speech (TTS) technology. This technology is an excellent example of assistive technology that has been developed to help individuals with visual impairments and those who have difficulty
HTML5 introduces the Speech API for AI Speech Synthesis and Speech Recognition. This is the easiest way to use the spoken word in your app or website. AI Speech Synthesis or more commonly known as Text To Speech (TTS) is now available in most modern browsers. Gone are the days of waiting for Text To Speech engines to render MP3 audio files from ...
I am looking for a good text to speech javascript library for my application. I looked around and saw Jtalk and speak.js. But I'm not sure which one to go forth with. ... My senior design project was for the blind, however we realized that the text to speech wasn't where we wanted to go because we felt Braille was better. With Braille you can ...