Verilog assign statement
Hardware schematic.
Signals of type wire or a similar wire like data type requires the continuous assignment of a value. For example, consider an electrical wire used to connect pieces on a breadboard. As long as the +5V battery is applied to one end of the wire, the component connected to the other end of the wire will get the required voltage.

In Verilog, this concept is realized by the assign statement where any wire or other similar wire like data-types can be driven continuously with a value. The value can either be a constant or an expression comprising of a group of signals.

Assign Syntax
The assignment syntax starts with the keyword assign followed by the signal name which can be either a single signal or a concatenation of different signal nets. The drive strength and delay are optional and are mostly used for dataflow modeling than synthesizing into real hardware. The expression or signal on the right hand side is evaluated and assigned to the net or expression of nets on the left hand side.
Delay values are useful for specifying delays for gates and are used to model timing behavior in real hardware because the value dictates when the net should be assigned with the evaluated value.
- LHS should always be a scalar or vector net or a concatenation of scalar or vector nets and never a scalar or vector register.
- RHS can contain scalar or vector registers and function calls.
- Whenever any operand on the RHS changes in value, LHS will be updated with the new value.
- assign statements are also called continuous assignments and are always active
In the following example, a net called out is driven continuously by an expression of signals. i1 and i2 with the logical AND & form the expression.
If the wires are instead converted into ports and synthesized, we will get an RTL schematic like the one shown below after synthesis.

Continuous assignment statement can be used to represent combinational gates in Verilog.
The module shown below takes two inputs and uses an assign statement to drive the output z using part-select and multiple bit concatenations. Treat each case as the only code in the module, else many assign statements on the same signal will definitely make the output become X.
Assign reg variables
It is illegal to drive or assign reg type variables with an assign statement. This is because a reg variable is capable of storing data and does not require to be driven continuously. reg signals can only be driven in procedural blocks like initial and always .
Implicit Continuous Assignment
When an assign statement is used to assign the given net with some value, it is called explicit assignment. Verilog also allows an assignment to be done when the net is declared and is called implicit assignment.
Combinational Logic Design
Consider the following digital circuit made from combinational gates and the corresponding Verilog code.
Combinational logic requires the inputs to be continuously driven to maintain the output unlike sequential elements like flip flops where the value is captured and stored at the edge of a clock. So an assign statement fits the purpose the well because the output o is updated whenever any of the inputs on the right hand side change.
After design elaboration and synthesis, we do get to see a combinational circuit that would behave the same way as modeled by the assign statement.

See that the signal o becomes 1 whenever the combinational expression on the RHS becomes true. Similarly o becomes 0 when RHS is false. Output o is X from 0ns to 10ns because inputs are X during the same time.
Click here for a slideshow with simulation example !
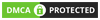
Using Continuous Assignment to Model Combinational Logic in Verilog
In this post, we talk about continuous assignment in verilog using the assign keyword. We then look at how we can model basic logic gates and multiplexors in verilog using continuous assignment.
There are two main classes of digital circuit which we can model in verilog – combinational and sequential .
Combinational logic is the simplest of the two, consisting solely of basic logic gates, such as ANDs, ORs and NOTs. When the circuit input changes, the output changes almost immediately (there is a small delay as signals propagate through the circuit).
In contrast, sequential circuits use a clock and require storage elements such as flip flops . As a result, output changes are synchronized to the circuit clock and are not immediate.
In this post, we talk about the techniques we can use to design combinational logic circuits in verilog. In the next post, we will discuss the techniques we use to model basic sequential circuits .
- Get the Video Tutorial
Purchase the video tutorial using the Fourdotpay Wallet.
Find the source code from this video tutorial on EDA Playground
We'd really appreciate your feedback on these videos and the Fourdotday payment portal. For feedback on the videos, please contact us on [email protected] . For feedback on the Fourdotpay platform, please contact us on [email protected] .
Payments for our videos are handled by a new company called Fourdotpay . Anyone who signs up with them during this introductory period will receive a £5 welcome bonus . This means you can try out one of our new videos absolutely free. See our FAQS page for details about how to take advantage of this offer.
Continuous Assignment in Verilog
We use continuous assignment to drive data onto verilog net types in our designs. As a result of this, we often use continuous assignment to model combinational logic circuits.
We can actually use two different methods to implement continuous assignment in verilog.
The first of these is known as explicit continuous assignment. This is the most commonly used method for continuous assignment in verilog.
In addition, we can also use implicit continuous assignment, or net declaration assignment as it is also known. This method is less common but it can allow us to write less code.
Let's look at both of these techniques in more detail.
- Explicit Continuous Assignment
We normally use the assign keyword when we want to use continuous assignment in verilog. This approach is known as explicit continuous assignment.
The verilog code below shows the general syntax for continuous assignment using the assign keyword.
The <variable> field in the code above is the name of the signal which we are assigning data to. We can only use continuous assignment to assign data to net type variables.
The <value> field can be a fixed value or we can create an expression using the verilog operators we discussed in a previous post. We can use either variable or net types in this expression.
When we use continuous assignment, the <variable> value changes whenever one of the signals in the <value> field changes state.
The code snippet below shows the most basic example of continuous assignment in verilog. In this case, whenever the b signal changes states, the value of a is updated so that it is equal to b.
- Net Declaration Assignment
We can also use implicit continuous assignment in our verilog designs. This approach is also commonly known as net declaration assignment in verilog.
When we use net declaration assignment, we place a continuous assignment in the statement which declares our signal. This can allow us to reduce the amount of code we have to write.
To use net declaration assignment in verilog, we use the = symbol to assign a value to a signal when we declare it.
The code snippet below shows the general syntax we use for net declaration assignment.
The variable and value fields have the same function for both explicit continuous assignment and net declaration assignment.
As an example, the verilog code below shows how we would use net declaration assignment to assign the value of b to signal a.
Modelling Combinational Logic Circuits in Verilog
We use continuous assignment and the verilog operators to model basic combinational logic circuits in verilog.
To show we would do this, let's look at the very basic example of a three input and gate as shown below.
To model this circuit in verilog, we use the assign keyword to drive the data on to the and_out output. This means that the and_out signal must be declared as a net type variable, such as a wire.
We can then use the bit wise and operator (&) to model the behavior of the and gate.
The code snippet below shows how we would model this three input and gate in verilog.
This example shows how simple it is to design basic combinational logic circuits in verilog. If we need to change the functionality of the logic gate, we can simply use a different verilog bit wise operator .
If we need to build a more complex combinational logic circuit, it is also possible for us to use a mixture of different bit wise operators.
To demonstrate this, let's consider the basic circuit shown below as an example.
To model this circuit in verilog, we need to use a mixture of the bit wise and (&) and or (|) operators. The code snippet below shows how we would implement this circuit in verilog.
Again, this code is relatively straight forward to understand as it makes use of the verilog bit wise operators which we discussed in the last post.
However, we need to make sure that we use brackets to model more complex logic circuit. Not only does this ensure that the circuit operates properly, it also makes our code easier to read and maintain.
Modelling Multiplexors in Verilog
Multiplexors are another component which are commonly used in combinational logic circuits.
In verilog, there are a number of ways we can model these components.
One of these methods uses a construct known as an always block . We normally use this construct to model sequential logic circuits, which is the topic of the next post in this series. Therefore, we will look at this approach in more detail the next blog post.
In the rest of this post, we will look at the other methods we can use to model multiplexors.
- Verilog Conditional Operator
As we talked about in a previous blog, there is a conditional operator in verilog . This functions in the same way as the conditional operator in the C programming language.
To use the conditional operator, we write a logical expression before the ? operator which is then evaluated to see if it is true or false.
The output is assigned to one of two values depending on whether the expression is true or false.
The verilog code below shows the general syntax which the conditional operator uses.
From this example, it is clear how we can create a basic two to one multiplexor using this operator.
However, let's look at the example of a simple 2 to 1 multiplexor as shown in the circuit diagram below.
The code snippet below shows how we would use the conditional operator to model this multiplexor in verilog.
- Nested Conditional Operators
Although this is not common, we can also write code to build larger multiplexors by nesting conditional operators.
To show how this is done, let's consider a basic 4 to 1 multiplexor as shown in the circuit below.
To model this in verilog using the conditional operator, we treat the multiplexor circuit as if it were a pair of two input multiplexors.
This means one multiplexor will select between inputs A and B whilst the other selects between C and D. Both of these multiplexors use the LSB of the address signal as the address pin.
To create the full four input multiplexor, we would then need another multiplexor.
This takes the outputs from the first two multiplexors and uses the MSB of the address signal to select between them.
The code snippet below shows the simplest way to do this. This code uses the signals mux1 and mux2 which we defined in the last example.
However, we could easily remove the mux1 and mux2 signals from this code and instead use nested conditional operators.
This reduces the amount of code that we would have to write without affecting the functionality.
The code snippet below shows how we would do this.
As we can see from this example, when we use conditional operators to model multiplexors in verilog, the code can quickly become difficult to understand. Therefore, we should only use this method to model small multiplexors.
- Arrays as Multiplexors
It is also possible for us to use verilog arrays to build simple multiplexors.
To do this we combine all of the multiplexor inputs into a single array type and use the address to point at an element in the array.
To get a better idea of how this works in practise, let's consider a basic four to one multiplexor as an example.
The first thing we must do is combine our input signals into an array. There are two ways in which we can do this.
Firstly, we can declare an array and then assign all of the individual bits, as shown in the verilog code below.
Alternatively we can use the verilog concatenation operator , which allows us to assign the entire array in one line of code.
To do this, we use a pair of curly braces - { } - and list the elements we wish to include in the array inside of them.
When we use the concatenation operator we can also declare and assign the variable in one statement, as long as we use a net type.
The verilog code below shows how we can use the concatenation operator to populate an array.
As verilog is a loosely typed language , we can use the two bit addr signal as if it were an integer type. This signal then acts as a pointer that determines which of the four elements to select.
The code snippet below demonstrates this method in practise. As the mux output is a wire, we must use continuous assignment in this instance.
What is the difference between implicit and explicit continuous assignment?
When we use implicit continuous assignment we assign the variable a value when we declare. When we use explicit continuous assignment we use the assign keyword to assign a value.
Write the code for a 2 to 1 multiplexor using any of the methods discussed we discussed.
Write the code for circuit below using both implicit and explicit continuous assignment.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Table of Contents
Sign up free for exclusive content.
Don't Miss Out
We are about to launch exclusive video content. Sign up to hear about it first.
L04 – Verilog Events, Timing, and Testbenches
1. references.
- (Schaumont 2010) Schaumont, Patrick
A Practical Introduction to Hardware/Software Codesign, Springer ISBN 978-1-4614-3737-6 https://www.springer.com/gp/book/9781441960009
2. Single Assignment Code
Consider the following code:
This is the same as
single assignment to a
can be implemented in hardware with
3. Conversion to Single Assignment Code
- ensure the in a pass of code a given variable is only assigned once
- introduction of new variAbles and
- renaming to ensure single assignments in a section of code.
- simplifies identification of the producing expression
- easier to understand the underlying data-flow
- data-dependency graphs can be generated more easily from single-assignment code
- unique identifiers facilitate association of hardware nets to variables in code
MERGE is a conceptual tool that can be introduced facilitate code analysis. We'll first motivate MERGE with an example.
Code with Loop/Branch
Conversion?
Solution is to use introduce the concept of a MERGE**. A MERGE maps to a mux in hardware, typically creating a loop or feedback.
Still must ensure that no combinatorial loops are formed, adding registers and multiple clock cycles as needed (below a2 may be implemented a register to ensure this).
!!! WARNING MERGE IS ONLY A CONCEPT * MERGE is a */concept/ , NOT an ACTUAL VERILOG LANGUAGE CONSTRUCT. Do not attempt to use merge in your code.
5. Unrolling and Simplification
Unrolling can be used to create single assignment code:
Algorithmic simplification , in this case arithmetic simplification can trim unnecessary complexity
6. Single Assignment Code Example
This example code describes an iterative algorithm that will be implemented with registers
Original Code
Single Assignment Code:
Hardware Implementation:

- Single Assignment Code allows examination of data dependencies and hardware resources such as what can be done in a single clock cycle (combinatorial) and where a register is required.
- These concepts are also important when writing behavioral HDL code in Verilog or VHDL.
7. Synthesizeable Combinatorial Code with attention to Loops
- Today we'll discuss a few constructs which involve a concern of Control Loops and Data (dependency) Loops in procedural HDL
- We'll first concern ourselves with combinatorial behavior and then allow additional flexibility with sequential
- Should not attempt to synthesize any code that implements unresolvable dependency loops as combinatorial HW (executes in one clock cycle)
8. Single Assignment Code
Think of each statement as a node on a graph with the edges denoting dependencies. Nodes can be producers and consumers of values. A graph with loops cannot be directly resolved as a combinatorial circuit. The inputs not generated from within the code are also nodes (they represent an assignment from elsewhere).
- Note how in this code segment alone the output is not well-defined. It is not clear of y1,y2 are used elsewhere or if they are just working variables for partial results.
Branches can be thought of as multiplexers that depend on the evaluation of conditional expression. A new flag variable resulting from the condition evaluation may be introduced to make this clear.
- To achieve the status of single assignment code, every variable may only be assigned once
- We may need to convert code to an equivalent single-assignment code to understand its underlying structure. To do this introduce additional variables when variables are assignment more than once
Original Code 1:
Single Assignment Code 1:
Original Code 2:
Single Assignment Code 2:
9. Externally formed feedback loops
- Consumption preceding production : If a procedural block consumes its own outputs before generating them, and external loop may be inferred
Some synthesis tools might produce an error and halt synthesis, but the SystemVerilog standard only states that
Software tools should perform additional checks to warn if the behavior within an always_comb procedure does not represent combinational logic, such as if latched behavior can be inferred.
An advanced code linter can find the issue:

Vivado 2023.2.2 exhibited no difference in behavior for always_comb vs. always @(*) in this code
- Inter-block combinational feedback : Combinational loops can be formed among multiple blocks, and this is to be avoided as well.
10. Verilog Synthesis: Feedback (data dependency loops)
It is important to be able to identify data dependency loops. Unresolvable loops cannot be implemented with combinational hardware.
- No feedback after substitutions
- Unresolveable Feedback

This clearly does not attempt to describe combinatorial hardware it uses an edge-selective trigger to describe sequential hardware. A register y is inserted by a synthesizer, but alongside other blocks, the danger is non-deterministic pre-synthesis simulation. This code should use non-blocking assignment.
11. A test for data dependency loops
- A conceptual test to understand if procedural code is implementing strictly combinatorial logic is to set every combinatorial result assigned to x at the entry point in the code, representing an erasure of any previous result lingering in the simulation
- If behavior would change in any case then code is not implementing strictly combinatorial logic
- ❌ when a : y='x
- ✔️combinatorial
- ❌ when a==1 : yA='x
- ❌ when a==1,b==1 : yA='x yB='x
- ❌ when a==1,b==1 : y='x
- ❌ when s : p='x
Remember, in pre-synthesis simulation you will see 1'bx as a result, but through synthesis the possibility of 1'bx is instead interpreted as a "don't care". If your code already achieved complete assignment without the initialization to 1b'x , then the initialization should have no affect.
‼️ Note 'x in Simulation vs. Synthesis 'x in a resulting in simulation output means that in simulation the value was undetermined. When a synthesizer analyzes code and determines that the code assigns 'x, it interprets it as a don't care and may optimizes according to implementation cost.
12. A test for implied loops in mixed logic blocks
- The combinational erasure test works for internal combinatorial values computed in edge-selective-trigger blocks.
- Setting combinatorial values to 'x at code entry may help identify issues in pre-synthesis simulation
- In the following code, does that combinatorial erasure have an effect?
❌ fails test
✔️ passes test
13. Unwanted Synthesized Cycle Iteration Loop (unintended cycle delay)
Figure 1: little feedback loop
Figure 2: no feedback loop
Module with Enable:
- The following modules produce the same result, with feedback from the register. Some give an explicit name to the net attached to the register's data input as _y.
✔️ explicit full specification of _y in each pass
Figure 3: explicit _y
❌ wrong style, even if result is the same memory inferred from _y rather than y is not proper
✔️ implied memory default for y is intended
✔️ in any given pass _y is only consumed after a production
14. Combinatorial Synthesis: Loops
We will classify traditionally coded loops in procedural code by how they are expected to be interpreted by synthesizers.
- Could directly perform finite loop unrolling
- Often synthesizers cannot convert non-static loops to combinatorial circuit.
- Dynamic-loops are those for which determination of the number of loop iterations required is determined at run-time, usually because the number of loops is determined by a run-time variable.
- In the example below, the condition that is checked before every iteration is dependent on assignments within the body of the loop.
Furthermore, the multiple data movements are problematic
While-like Loop:
The previous code can be rewritten to have well-defined static loop count and no implied data movement. The constant 8 sets the statically defined loop count.
!!! Tip What can and cannot be synthesized depends on the synthesizer The logic function is not fundamentally excluded from mapping to cominational hardware, as seen in the next example. So, while this code might not be synthesizable by every synthesizer today, others might be able to analyze the code and interpret the combinational hardware function successfully. Also what a given synthesizer can't do today, it may be able to do tomorrow. Your synthesizer should provide documentation on what constructs are allowed and it is worth checking yearly.
15. Synthesis: Feedback (data dependency loops)
Registered output logic (mix comb and seq.) should be separated to understand the dependencies. New variables may be introduced to denote the difference in signals before and after a register.
Feedback is perhaps unclear here. See rewrite below.
Figure 4: counter
- Feedback across clock cycles is acceptable.
- No feedback in combinational partition.
16. Synthesis: keyword disable
- The keyword disable may be used to implement a "break" from a loop. Consider this not yet covered and avoid for now if without further study.
17. Sequential Synthesis: Multi-Cycle Loops
Note that you may be able describe a sequential circuit with non-static loops, but it is common to be NOT SUPPORTED by synthesizers. The following suggests a multi-cycle (multiple clock periods) operation.
"while" loop with iteration synced to a clock:
18. Synthesis: Multi-cycle Operations
- This is a forward note, we'll learn about this more later in the course.
It is typical to employ multi-cycle operations to reduce hardware through resource sharing (reuse of hardware in difference clock cycles) and reduce the critical path lengths.
Implicit state machines to describe multi-cycle operations are not supported for synthesis: In the land of simulation one can model such behavior as follows, though it is usually not supported for synthesis
- We'll want to understand how to implement multi-cycle operations using state machines to create variations based on the number of resources (e.g. multipliers, registers) affordable or desired, as factors like clock-cycle latency and throughput, power, clock freq., etc…
!!! Warning Multi-cycle computations Multi-cycle computations and rarely supported constructs above are shown here for completeness. Later lectures will formally introduce methods for such descriptions. Do not attempt to use these in synthesizable code without further study.
19. Key Points pertaining to Loops
- Combinational hardware with data dependency loops cannot be synthesized
- Static for-loops can be synthesized by being unrolled
- Dynamic for-loops might not be understood by the synthesizer
- Dynamic for-loops with timing control might be synthesized as a "multicycle operation" or a state machine, but many synthesizers do not support this construct
- We'll want to formalize multi-cycle operations as state machines a little later in this course.

20. Generate Constructs (structural for loops, and conditional structuals)
- Outside procedural blocks, keywords for and if are supported
:CUSTOM ID : RANDOM-ID-catjy
structural "for loop": for-in-generate
- Uses a special indexing variable defined using genvar. Within a generate block indicated using keyword generate
Use for repetitive structural instantiations
for-in-generate example: Adder
An adder is a good example since it involves a repetitive structure with interconnection between instantiations (/use for param) / Study the details of the carefully crafted index in the carry out of each instance
- ⭐ Note Updated Syntax in SystemVerilog: In SystemVerilog the generate and endgenerate keywords are optional . Under many use cases, those keywords could be obviously inferred by the interpreter, such as when the for loop utilizes a genvar variable, and thus the additional keywords are superfluous.
- use label on begin...end block , such as some_hierarchical_label in the previous example.
- Variables and instances defined within can be accessed with the hierarchy <parent>.<some_hierarchical_label>.<children> or in the case of for loops: <parent>.some_some_hierarchical_label[<generation_index>].<children>
inferred hierarchical names from conditions : In SystemVerilog, names for conditional statements are implied for unnamed if blocks if involving simple named parameter checking
21. Concluding Points
- Combinatorial dependency loops cannot be synthesized
- Static for loops can be synthesized by being unrolled
- Dynamic for Loops may not be synthesizable
- Dynamic for Loops with timing control may be synthesized as a "multicycle operation" or a state machine.
- Repetitive/Patterned Structural Instantiations may be done with for…generate loops
- We'll want to formalize multi-cycle operations as state machines.
22. Interesting Synthesis Example of Control Signals and Data
A conditional addition such as
are often synthesized with an adder, as in
simplifying the circuity.
23. Example Exercise and Discussion on Static/Dynamic Loop Determination
Does the for loop below represent a dynamic or static loop?
Hint1: Start by trying to unroll the loop iterations
Where to stop is not obvious until a is known, which only happens at "run-time"
How to make the loop static?
Proposal 1: set a to a fixed value? * this would make the loop static but since a is an input from another module we want to keep the input
Next Hint: Can you predetermine the possible range of i , which is based on the range of a ?
Lets try to unroll the loop starting with the first iteration i=0.
i=0 involves setting thermocode[0]
When is thermocode[0] set to 1?
Ans: when a>0, resulting in thermocode[0]=a>0
We can realize that though a can go up to 29, the thermocode only includes 30 bits
Now, lets shorthand this long set of repetitive code as a static loop:
- So, should the original code be considered "synthesizable" or not?
- depends on the synthesizer. The context in which the loop exists (here the context involves a bounded a ) may be of importance, but may or may not be taken into account by the synthesizer.
- Increasingly smart synthesizers can synthesize dynamic loops, but the designer should understand the equivalent static loop and the relationship to hardware.
Author: Dr. Ryan Robucci
Created: 2024-09-19 Thu 13:55
Modeling Concurrent Functionality in Verilog
- First Online: 01 March 2019
Cite this chapter
- Brock J. LaMeres 2
84k Accesses
This chapter presents a set of built-in operators that will allow basic logic expressions to be modeled within a Verilog module. This chapter then presents a series of combinational logic model examples.
This is a preview of subscription content, log in via an institution to check access.
Access this chapter
Subscribe and save.
- Get 10 units per month
- Download Article/Chapter or eBook
- 1 Unit = 1 Article or 1 Chapter
- Cancel anytime
- Available as PDF
- Read on any device
- Instant download
- Own it forever
- Available as EPUB and PDF
Tax calculation will be finalised at checkout
Purchases are for personal use only
Institutional subscriptions
Author information
Authors and affiliations.
Department of Electrical & Computer Engineering, Montana State University, Bozeman, MT, USA
Brock J. LaMeres
You can also search for this author in PubMed Google Scholar
Rights and permissions
Reprints and permissions
Copyright information
© 2019 Springer Nature Switzerland AG
About this chapter
LaMeres, B.J. (2019). Modeling Concurrent Functionality in Verilog. In: Quick Start Guide to Verilog. Springer, Cham. https://doi.org/10.1007/978-3-030-10552-5_3
Download citation
DOI : https://doi.org/10.1007/978-3-030-10552-5_3
Published : 01 March 2019
Publisher Name : Springer, Cham
Print ISBN : 978-3-030-10551-8
Online ISBN : 978-3-030-10552-5
eBook Packages : Engineering Engineering (R0)
Share this chapter
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Publish with us
Policies and ethics
- Find a journal
- Track your research
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Why can't regs be assigned to multiple always blocks in synthesizable Verilog?
The accepted answer to this question notes that "every reg variable can only be assigned to in at most one always statement". It's clear that in a lot of cases assigning a reg to multiple always blocks is meaningless. However, it's seems that there could be hardware-meaning instances of a reg in different always blocks.
For example, what if a same reg is assigned to always @(posedge clk1) , always @(posedge clk2) where clk1 , clk2 never beat of the same time? There would be no race-condition.
Why is there a "hard rule" concerning reg s in different always blocks?
2 Answers 2
For example, what if a same reg is assigned to always @(posedge clk1), always @(posedge clk2) where clk1, clk2 never beat of the same time? There would be no race-condition.
The case you mention will not be synthesizable. The flip-flop that implements the reg can only be clocked from one source.
As an aside, I'd like to clarify that the rule about reg s having affinity to always blocks affects synthesizability, not validity. Software simulator will handle assignments from different always blocks without any problems.
- \$\begingroup\$ What about always @(posedge clk), always @(negedge clk) ? \$\endgroup\$ – Rocketmagnet Commented Apr 10, 2012 at 10:10
- 1 \$\begingroup\$ @Rocketmagnet, that's the same problem. Flip-flops react to one of the edges. \$\endgroup\$ – avakar Commented Apr 10, 2012 at 10:18
- \$\begingroup\$ DDR flip-flops (available in IO blocks of many FPGAs) react to both edges. But even then, there's only one input, so it doesn't make sense to have the logic split up into multiple blocks. always @(posedge clk or negedge clk) or just always @clk would make sense. Even so, I'm not sure if any tools will synthesize those -- I have always explicitly instantiated the library block instead. \$\endgroup\$ – The Photon Commented Apr 10, 2012 at 15:36
- \$\begingroup\$ @ThePhoton, that is my experience as well with Altera FPGAs: special peripherals (including DDR transceivers) are black-box modules, which can be instantiated into your design. \$\endgroup\$ – avakar Commented Apr 10, 2012 at 15:51
Although you may know that two clock edges will never arrive simultaneously, the synthesizer can't assume that. It's possible to construct a reasonable-cost circuit which will latch D1 on the rising edge of C1, and D2 on the rising edge of C2, provided that rising edges of C1 and C2 are always sufficiently separated. On the other hand, a pair of "always" blocks would indicate that such a circuit should also correctly and without glitches handle the case where the latch start out low, D1 and D2 are both high, and C1 and C2 arrive in arbitrary sequence, with arbitrary time between them. Even if a synthesizer could generate such a thing, the circuit cost would be more than double what would be needed to handle the case where C1 and C2 are always disjoint.
Your Answer
Sign up or log in, post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged verilog or ask your own question .
- The Overflow Blog
- Looking under the hood at the tech stack that powers multimodal AI
- Detecting errors in AI-generated code
- Featured on Meta
- User activation: Learnings and opportunities
- Preventing unauthorized automated access to the network
Hot Network Questions
- Numerical integration of ODEs: Why does higher accuracy and precision not lead to convergence?
- Is it possible to make sand from bones ? Would it have the same properties as regular sand?
- Does Psalm 56:8 speak of 'measuring of prayers ' by God?
- Can't redeem my denied-boarding vouchers
- How to prevent leaves from going into the water drains of a flat roof?
- Why is it surprising that the CMB is so homogeneous?
- Why is angular momentum in a 2-body system conserved if the points about which we take them are moving?
- How do cafes prepare matcha in a foodsafe way, if a bamboo whisk/chasen cannot be sanitized in a dishwasher?
- Terminated employee will not help the company locate its truck
- Which tool has been used to make this puzzle?
- SF story set in an isolated (extragalactic) star system
- Returning to the US for 2 weeks after a short stay around 6 months prior with an ESTA but a poor entry interview - worried about visiting again
- Is it possible to monitor the current drawn by a computer from an outlet on the computer?
- Can there be a proper class of Dedekind-finite cardinals?
- Is the ind-completion of a triangulated category triangulated?
- CC BY-SA 2.5 License marked as denied license in the FOSSA tool after upgrading to React Native 0.74 version
- How to know what to insert in the TO SHIFT in airbus MCDU?
- Convert polygon geometry to renderable hair with Geometry Nodes
- Crime and poverty: Correlation or causation?
- Quote about expecting robots to take our manual labour from us but now AI is taking our creativity instead?
- Relational-join Excel tables
- Why are Jesus and Satan both referred to as the morning star?
- Why does lottery write "in trust" on winner's cheque?
- Has the UN ever made peace between two warring parties?
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Multiple conditions in If statement Verilog
I have the following if/else statement :
I am getting these errors :
So what is the problem here ?

2 Answers 2
You have a space in your variable name.
Note this occurs twice - in the “if” and the first “else if”
For someone who stumbles upon this question looking for a syntax reference, following are the excerpts from the sections " 4.1.9 Logical operators " and " 9.4 Conditional statement " in one of the revisions of the Verilog standard .
This is syntax of if statement :
If the expression evaluates to true (that is, has a nonzero known value), the first statement shall be executed. If it evaluates to false (has a zero value or the value is x or z ), the first statement shall not execute. If there is an else statement and expression is false, the else statement shall be executed.
And this is about logical operators to combine multiple conditions in one expression:
The operators logical and ( && ) and logical or ( || ) are logical connectives. The result of the evaluation of a logical comparison shall be 1 (defined as true ), 0 (defined as false ), or, if the result is ambiguous, the unknown value ( x ). The precedence of && is greater than that of || , and both are lower than relational and equality operators. A third logical operator is the unary logical negation operator ( ! ). The negation operator converts a nonzero or true operand into 0 and a zero or false operand into 1 .
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged verilog or ask your own question .
- The Overflow Blog
- Looking under the hood at the tech stack that powers multimodal AI
- Detecting errors in AI-generated code
- Featured on Meta
- User activation: Learnings and opportunities
- Preventing unauthorized automated access to the network
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- How to know what to insert in the TO SHIFT in airbus MCDU?
- How to prevent leaves from going into the water drains of a flat roof?
- What effect will a planet’s narcotic atmosphere have on sound of music at rave parties?
- Buying property in Switzerland
- What happened to the periodic scripts on macOS Sequoia?
- Why is it surprising that the CMB is so homogeneous?
- How Does God Feel Love For His Creations?
- Why are no metals green or blue?
- Enter a personal identification number
- Does it ever make sense to have a one-to-one obligatory relationship in a relational database?
- the usage of phrase 'Leave ... behind'
- Which tool has been used to make this puzzle?
- If given 3 random real numbers between 0-100, what is the probability that the median is closest in value to 50?
- Uppercase, lowercase – "in any case"?
- BSS138 level shifter - blocking current flow when high-side supply is not connected?
- Is it possible to make sand from bones ? Would it have the same properties as regular sand?
- Is it possible to monitor the current drawn by a computer from an outlet on the computer?
- Terrible face recognition skills: how to improve
- How does NASA calculate trajectory at planetary atmosphere entry
- Why do you even need a heatshield - why not just cool the re-entry surfaces from inside?
- Will running a dehumidifier in a basement impact room temperature?
- Why is the #16 always left open?
- How do I reposition lag screws in garage after drywall is up?
- Is "Canada's nation's capital" a mistake?

IMAGES
VIDEO
COMMENTS
The LHS of an assign statement cannot be a bit-select, part-select or an array reference but can be a variable or a concatenation of variables. reg q; initial begin assign q = 0; #10 deassign q; end force release. These are similar to the assign - deassign statements but can also be applied to nets and variables. The LHS can be a bit-select of ...
2. If all the variables have the same bit-width, and therefore the values you want assigned to those variables have the same bit-width, you can do a replication concatenation: {a,b,c,d,e} = {5{value}}; answered Aug 4, 2017 at 23:13. dave_59.
Verilog assign statement. Signals of type wire or a similar wire like data type requires the continuous assignment of a value. For example, consider an electrical wire used to connect pieces on a breadboard. As long as the +5V battery is applied to one end of the wire, the component connected to the other end of the wire will get the required ...
Recent versions of Verilog provides a means to implement the sensitivity list without explicitly listing each potential variable. Instead of listing variables as in the previous example always @ (a or b or sel) Simply use always @*. The * operator will automatically identify all sensitive variables.
In VHDL, when you have multiple assignments to a signal within a process (i.e. an always block), whether it's sequential or combinatorial, the signal assignment that's written last in the code will take precedence. Since you're assigning signals, not variables, the right-hand side value of count in the enable2 block is the value it had in the ...
You can only have one source driving a given net, so having result being assigned in multiple places won't work (for synthesis at least). Furthermore the output a module cannot be connected directly to a reg type variable. Instead, to connect multiple things together in Verilog, you use wire (net data type). For example
The first verilog example does the job but it takes a bit to understand the logic. Please keep in mind I am not complaining about or criticizing any language, I just want to know how to improve code readability for this particular case.
Multiple continuous assignments can be made to the same net. When this happens, the assignment containing signals with the highest drive strength will take priority. Example: assign F1 = A; ... Below is another example of how continuous signal assignments in Verilog differ from a sequentially executed programming language. Example:
Verilog concurrent assignment to register. Ask Question. Asked 4 years, 4 months ago. Modified 4 years, 4 months ago. Viewed 434 times.
In this post, we talk about continuous assignment in verilog using the assign keyword. We then look at how we can model basic logic gates and multiplexors in verilog using continuous assignment.. There are two main classes of digital circuit which we can model in verilog - combinational and sequential. Combinational logic is the simplest of the two, consisting solely of basic logic gates ...
of other Verilog nonblocking assignments can also be evaluated and LHS updates scheduled. The nonblocking assignment does not block other Verilog statements from being evaluated. Execution of nonblocking assignments can be viewed as a two-step process: 1. Evaluate the RHS of nonblocking statements at the beginning of the time step. 2.
The recommended Verilog coding practice is to always use non-blocking assignments to describe sequential logic. This means that assignments to signals inside an always procedural block which has a posegde clk (or similar) in the sensitivity list should use <=, not =. Doing so leads to predictable simulation results, both before and after synthesis.
when s: p='x; Remember, in pre-synthesis simulation you will see 1'bx as a result, but through synthesis the possibility of 1'bx is instead interpreted as a "don't care". If your code already achieved complete assignment without the initialization to 1b'x, then the initialization should have no affect.. ‼️ Note 'x in Simulation vs. Synthesis 'x in a resulting in simulation output means ...
Function return values can be specified in two ways, either by using a return statement or by assigning a value to the internal variable with the same name as the function. This seems a little unclear as to whether you can assign multiple times, like in my example. Furthermore, the example from the LRM directly after this statement and also all ...
3.1.1 Assignment Operator. Verilog uses the equal sign (1⁄4) to denote an assignment. The left-hand side (LHS) of the assign-ment is the target signal. The right-hand side (RHS) contains the input arguments and can contain both signals, constants, and operators. Example: F1 A; // F1 is assigned the signal A.
The accepted answer to this question notes that "every reg variable can only be assigned to in at most one always statement". It's clear that in a lot of cases assigning a reg to multiple always blocks is meaningless. However, it's seems that there could be hardware-meaning instances of a reg in different always blocks.. For example, what if a same reg is assigned to always @(posedge clk1 ...
If it evaluates to false (has a zero value or the value is x or z), the first statement shall not execute. If there is an else statement and expression is false, the else statement shall be executed. And this is about logical operators to combine multiple conditions in one expression: The operators logical and (&&) and logical or (||) are ...